帮我写一个简易的pythonFTP客户端
时间: 2023-09-11 18:04:47 浏览: 83
### 回答1:
好的,下面是一个简单的Python FTP客户端,用于连接FTP服务器并执行一些基本操作:
```python
import ftplib
# 连接FTP服务器
ftp = ftplib.FTP("ftp.example.com")
ftp.login("username", "password")
# 列出FTP服务器上的文件
print("FTP服务器上的文件列表:")
ftp.dir()
# 下载文件
filename = "example.txt"
with open(filename, "wb") as f:
ftp.retrbinary("RETR " + filename, f.write)
# 上传文件
filename = "example.txt"
with open(filename, "rb") as f:
ftp.storbinary("STOR " + filename, f)
# 删除文件
filename = "example.txt"
ftp.delete(filename)
# 关闭连接
ftp.quit()
```
请注意,这只是一个简单的示例,实际上您可能需要进行更多的错误处理和用户输入验证。
### 回答2:
Python中的`ftplib`模块提供了一个用于编写FTP客户端的接口。你可以使用这个模块编写一个简易的Python FTP客户端。
下面是一个简单的例子,展示了如何使用`ftplib`模块连接到FTP服务器、进行文件上传和下载等操作:
```python
import ftplib
def ftp_upload(hostname, username, password, local_file, remote_file):
try:
ftp = ftplib.FTP(hostname)
ftp.login(username, password)
with open(local_file, 'rb') as file:
ftp.storbinary(f'STOR {remote_file}', file)
print('文件上传成功!')
except ftplib.all_errors as e:
print(f'文件上传失败:{e}')
def ftp_download(hostname, username, password, remote_file, local_file):
try:
ftp = ftplib.FTP(hostname)
ftp.login(username, password)
with open(local_file, 'wb') as file:
ftp.retrbinary(f'RETR {remote_file}', file.write)
print('文件下载成功!')
except ftplib.all_errors as e:
print(f'文件下载失败:{e}')
# 使用示例
hostname = 'ftp.example.com'
username = 'your-username'
password = 'your-password'
local_file = 'local-file.txt'
remote_file = 'remote-file.txt'
# 上传文件
ftp_upload(hostname, username, password, local_file, remote_file)
# 下载文件
ftp_download(hostname, username, password, remote_file, local_file)
```
你需要将`hostname`、`username`、`password`、`local_file`和`remote_file`替换成你要连接的FTP服务器的信息和操作的文件信息。
这个例子只是一个简单的FTP客户端,你可以根据需要进行扩展。你可以使用`ftplib`模块提供的其他方法,例如列出目录内容、删除文件等等。此外,你还可以使用`os`模块等相关模块对文件进行进一步的处理。
### 回答3:
当然可以帮你写一个简易的Python FTP客户端。以下是一个使用Python的ftplib模块的示例:
```python
import os
from ftplib import FTP
def ftp_upload(hostname, username, password, local_dir, remote_dir):
try:
ftp = FTP(hostname)
ftp.login(username, password)
ftp.cwd(remote_dir)
for filename in os.listdir(local_dir):
local_path = os.path.join(local_dir, filename)
if os.path.isfile(local_path):
with open(local_path, 'rb') as file:
ftp.storbinary('STOR ' + filename, file)
ftp.quit()
print("文件上传完成!")
except Exception as e:
print("文件上传失败!错误信息:", str(e))
def ftp_download(hostname, username, password, remote_dir, local_dir):
try:
ftp = FTP(hostname)
ftp.login(username, password)
ftp.cwd(remote_dir)
filenames = ftp.nlst()
for filename in filenames:
local_path = os.path.join(local_dir, filename)
with open(local_path, 'wb') as file:
ftp.retrbinary('RETR ' + filename, file.write)
ftp.quit()
print("文件下载完成!")
except Exception as e:
print("文件下载失败!错误信息:", str(e))
# 使用示例
host = 'ftp.example.com'
username = 'your_username'
password = 'your_password'
local_directory = 'C:/local_dir' # 本地目录
remote_directory = '/remote_dir' # 远程目录
ftp_upload(host, username, password, local_directory, remote_directory) # 上传文件
ftp_download(host, username, password, remote_directory, local_directory) # 下载文件
```
上述代码使用ftplib模块中的FTP类,实现了上传和下载文件的功能。你需要根据你的FTP服务器信息,提供正确的主机名、用户名和密码。同时,你需要提供本地目录和远程目录,来指定文件的上传和下载路径。代码中使用的是绝对路径,请根据你自己的需求进行修改。
相关推荐
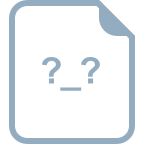
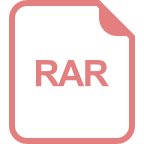














