聊天软件中,用Java实现消息从输入框中发送出去显示在消息面板后输入框内容清空的功能
时间: 2024-02-18 16:04:25 浏览: 25
要实现这个功能,可以使用Java Swing框架中的JTextField和JTextArea组件。
首先,创建一个JTextField组件来接收用户输入的消息,然后添加一个ActionListener监听器,当用户按下“发送”按钮或按下回车键时,触发事件。
在事件处理程序中,获取输入框中的文本,将其添加到JTextArea组件中,并清空输入框内容。
以下是示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ChatApp extends JFrame {
private JTextField textField;
private JTextArea textArea;
public ChatApp() {
super("Chat App");
// 创建输入框和消息面板
textField = new JTextField(20);
textArea = new JTextArea(10, 20);
textArea.setEditable(false);
// 将输入框添加到窗口
add(textField, BorderLayout.NORTH);
// 将消息面板添加到滚动窗格,并将其添加到窗口
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// 添加事件监听器
textField.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// 将输入框中的文本添加到消息面板
textArea.append(textField.getText() + "\n");
// 清空输入框
textField.setText("");
}
});
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public static void main(String[] args) {
new ChatApp();
}
}
```
在这个例子中,我们创建了一个窗口,包含一个输入框和一个消息面板。当用户在输入框中输入文本并按下回车键时,我们将文本添加到消息面板中,并清空输入框。通过使用JScrollPane组件,我们可以使消息面板具有滚动条,以便用户可以查看以前发送的消息。
相关推荐
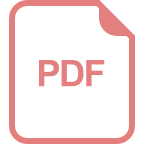
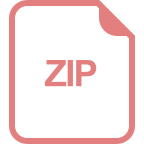














