使用C语言写一个基于lpc214 的直流电机控制系统,系统要求用pid控制算法
时间: 2024-03-10 20:44:40 浏览: 91
以下是一个基于lpc214的直流电机控制系统的代码示例,使用PID控制算法:
```c
#include <LPC214x.h>
#include <stdint.h>
#define PWM_MATCH_CHANNEL 0
#define PWM_PERIOD 1000
volatile uint32_t pwm_pulse_width = 0;
volatile uint32_t speed_setpoint = 0;
volatile uint32_t speed_feedback = 0;
volatile uint32_t error_integral = 0;
void PWM0_Init(void)
{
// Enable PWM0 peripheral clock
PCONP |= (1 << 6);
// Configure PWM0 peripheral clock
PCLKSEL0 &= ~(3 << 12);
PCLKSEL0 |= (1 << 12);
// Configure PWM0 pins for output
PINSEL0 |= (1 << 21);
// Configure PWM0 peripheral
PWM0TCR = (1 << 1);
PWM0PR = 0;
PWM0MCR = (1 << 1);
PWM0PCR = (1 << 9);
PWM0MR0 = PWM_PERIOD;
PWM0MR1 = pwm_pulse_width;
PWM0LER = (1 << PWM_MATCH_CHANNEL);
PWM0TCR = (1 << 0) | (1 << 3);
}
void ADC_Init(void)
{
// Enable ADC peripheral clock
PCONP |= (1 << 12);
// Configure ADC peripheral clock
PCLKSEL0 &= ~(3 << 24);
PCLKSEL0 |= (1 << 24);
// Configure ADC pins for input
PINSEL1 |= (1 << 18);
// Configure ADC peripheral
ADCR = (1 << 21) | (1 << 8) | (1 << 0);
}
uint32_t ADC_Read(void)
{
ADCR |= (1 << 24);
while (!(ADGDR & (1 << 31)));
return (ADGDR >> 4) & 0xfff;
}
void delay_ms(uint32_t ms)
{
uint32_t i, j;
for (i = 0; i < ms; i++)
{
for (j = 0; j < 1000; j++);
}
}
void PID_Controller(void)
{
static uint32_t error_previous = 0;
static uint32_t pwm_pulse_width_previous = 0;
uint32_t error = speed_setpoint - speed_feedback;
error_integral += error;
if (error_integral > 100)
{
error_integral = 100;
}
else if (error_integral < -100)
{
error_integral = -100;
}
uint32_t error_derivative = error - error_previous;
pwm_pulse_width = pwm_pulse_width_previous + error * 0.5 + error_integral * 0.2 + error_derivative * 0.3;
if (pwm_pulse_width > PWM_PERIOD)
{
pwm_pulse_width = PWM_PERIOD;
}
else if (pwm_pulse_width < 0)
{
pwm_pulse_width = 0;
}
error_previous = error;
pwm_pulse_width_previous = pwm_pulse_width;
}
int main()
{
PWM0_Init();
ADC_Init();
while (1)
{
uint32_t adc_value = ADC_Read();
speed_feedback = adc_value * 100 / 0xfff;
PID_Controller();
PWM0MR1 = pwm_pulse_width;
PWM0LER = (1 << PWM_MATCH_CHANNEL);
delay_ms(10);
}
}
```
这个示例代码是一个基于lpc214的直流电机控制系统,使用了PID控制算法来控制电机的转速。在每次循环中,读取ADC输入值,计算电机实际转速,并使用PID控制算法计算出PWM输出占空比。控制系统将持续地读取ADC输入,并根据输入值和设定值调整PWM输出,从而控制电机的转速和转矩。
阅读全文
相关推荐
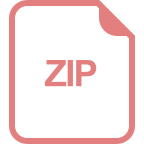
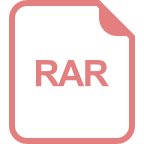
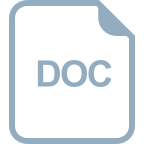
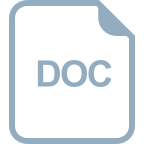
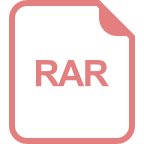
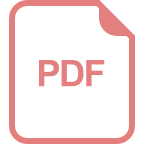
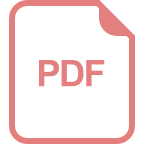
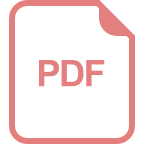
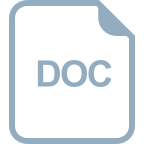
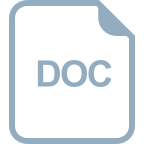
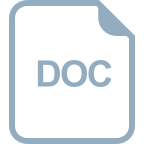
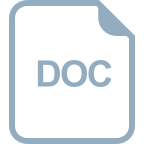
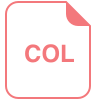
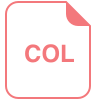
