linux通过I/O操作创建文件并复制另一个文件内容到该文件
时间: 2023-07-31 08:11:08 浏览: 57
您可以使用Linux系统调用中的open、read、write和close等函数来进行文件操作。具体步骤如下:
1. 使用open函数创建一个新的文件,如果文件已经存在则覆盖原有文件。例如:
```
int fd = open("new_file.txt", O_CREAT | O_WRONLY | O_TRUNC, 0644);
```
2. 使用open函数打开需要复制内容的文件,例如:
```
int fd2 = open("old_file.txt", O_RDONLY);
```
3. 使用read函数读取需要复制的文件的内容,例如:
```
char buf[1024];
int n = read(fd2, buf, sizeof(buf));
```
4. 使用write函数将读取到的内容写入新的文件中,例如:
```
write(fd, buf, n);
```
5. 关闭文件,例如:
```
close(fd);
close(fd2);
```
完整的代码示例如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd, fd2;
char buf[1024];
int n;
// 创建新文件
fd = open("new_file.txt", O_CREAT | O_WRONLY | O_TRUNC, 0644);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 打开需要复制的文件
fd2 = open("old_file.txt", O_RDONLY);
if (fd2 == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 读取需要复制的文件的内容,并写入新文件中
while ((n = read(fd2, buf, sizeof(buf))) > 0) {
write(fd, buf, n);
}
// 关闭文件
close(fd);
close(fd2);
return 0;
}
```
注意,以上代码仅供参考,实际应用中还需要添加错误处理等完善的内容。
相关推荐
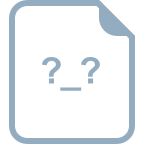
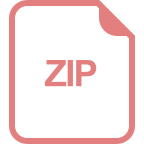
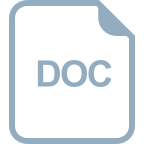














