#include <stdio.h> #include <pthread.h> #include <semaphore.h> #include <stdlib.h> #include <string.h> #define BUFFER_SIZE 200 char* buffer;//缓冲区 sem_t empty_sem;//空缓冲区信号量 sem_t full_sem;//满缓冲区信号量 pthread_mutex_t mutex;//互斥信号量 void *producer(void *arg) { // 等待空缓冲区 sem_wait(&empty_sem); pthread_mutex_lock(&mutex); // 将产品放入缓冲区 printf("input sonething to buffer:"); fgets(buffer,BUFFER_SIZE,stdin); pthread_mutex_unlock(&mutex); sem_post(&full_sem); pthread_exit(NULL); } void *consumer(void *arg) { // 等待满缓冲区 sem_wait(&full_sem); pthread_mutex_lock(&mutex); // 从缓冲区取出产品 printf("read product from buffer:%s", buffer); memset(buffer,0,BUFFER_SIZE); pthread_mutex_unlock(&mutex); sem_post(&empty_sem); pthread_exit(NULL); } int main(){ pthread_t tid1,tid2; buffer=(char*)malloc(BUFFER_SIZE); //初始化信号量和互斥信号量 sem_init(&empty_sem,0,BUFFER_SIZE);//初始化empty_sem的值为BUFFER_SIZE sem_init(&full_sem,0,0); pthread_mutex_init(&mutex,NULL); //创建生产者和消费者线程 pthread_create(&tid1,NULL,producer,NULL); pthread_create(&tid2,NULL,consumer,NULL); //等待线程结束 pthread_join(tid1,NULL); pthread_join(tid2,NULL); //销毁信号量和互斥信号量 sem_destroy(&empty_sem); sem_destroy(&full_sem); pthread_mutex_destroy(&mutex); printf("The End!\n"); return 0; }
时间: 2024-04-06 15:30:56 浏览: 118
这是一个生产者-消费者问题的代码实现,其中使用互斥量和信号量来实现线程同步和互斥。生产者线程等待空缓冲区信号量,然后获取互斥信号量,将产品放入缓冲区,释放互斥信号量,然后发出满缓冲区信号量。消费者线程等待满缓冲区信号量,然后获取互斥信号量,从缓冲区取出产品,释放互斥信号量,然后发出空缓冲区信号量。主函数中创建生产者和消费者线程,并等待它们结束。在程序结束时,销毁互斥量和信号量。
相关问题
优化这段代码//为消息发送程序 #include<stdio.h> #include<stdlib.h> #include<string.h> #include<sys/stat.h> #include<fcntl.h> #include<pthread.h> #include<semaphore.h> #include<sys/types.h> #include<unistd.h> #include<sys/ipc.h> #include<sys/shm.h> static const char * MUTEX_NAME = "mutex_shm"; static const char * FULL_NAME = "full_shm"; #define INPUT_SIZE 1024 //输入的最大长度 #define KEY_NUM 8848 void P(sem_t *semPtr){ sem_wait(semPtr); } void V(sem_t *semPtr){ sem_post(semPtr); } int main(int argc, char** argv){ key_t key = KEY_NUM; //为共享内存段命名 char input[INPUT_SIZE]; char reply[INPUT_SIZE]; int shmid; char* shmptr; //创建共享内存 shmid = shmget(key, INPUT_SIZE, IPC_CREAT | 0666); if(shmid < 0) { perror("Receiver: Shmget Error"); exit(EXIT_FAILURE); } //启动对该共享内存的访问,并把共享内存连接到当前进程的地址空间 shmptr = shmat(shmid, NULL, 0); sem_t* mutex = sem_open(MUTEX_NAME,O_CREAT); //共享内存只能同时一个程序访问 sem_t* full = sem_open(FULL_NAME,O_CREAT); //共享内存的消息数量 printf("请输入一串字符:"); scanf("%s",input); P(mutex); strcpy(shmptr,input); V(mutex); V(full); printf("消息已发送给receiver!\n"); //把共享内存从当前进程中分离 if(shmdt(shmptr) == -1){ fprintf(stderr, "shmdt failed\n"); exit(EXIT_FAILURE); } return 0; }
这段代码可以进行以下优化:
1. 在创建信号量时,应该设置初始值,否则可能会出现死锁的情况。
2. 在使用信号量时,应该先判断是否成功获取信号量,否则可能会出现程序崩溃的情况。
3. 应该检查共享内存的连接是否成功。
4. 在结束程序之前,应该关闭信号量和共享内存。
下面是优化后的代码:
```
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/stat.h>
#include<fcntl.h>
#include<pthread.h>
#include<semaphore.h>
#include<sys/types.h>
#include<unistd.h>
#include<sys/ipc.h>
#include<sys/shm.h>
static const char * MUTEX_NAME = "mutex_shm";
static const char * FULL_NAME = "full_shm";
#define INPUT_SIZE 1024 //输入的最大长度
#define KEY_NUM 8848
void P(sem_t *semPtr){
if(sem_wait(semPtr) != 0){
perror("P: sem_wait() failed");
exit(EXIT_FAILURE);
}
}
void V(sem_t *semPtr){
if(sem_post(semPtr) != 0){
perror("V: sem_post() failed");
exit(EXIT_FAILURE);
}
}
int main(int argc, char** argv){
key_t key = KEY_NUM; //为共享内存段命名
char input[INPUT_SIZE];
int shmid;
char* shmptr;
sem_t* mutex;
sem_t* full;
//创建共享内存
shmid = shmget(key, INPUT_SIZE, IPC_CREAT | 0666);
if(shmid < 0)
{
perror("Receiver: Shmget Error");
exit(EXIT_FAILURE);
}
//启动对该共享内存的访问,并把共享内存连接到当前进程的地址空间
shmptr = shmat(shmid, NULL, 0);
if(shmptr == (char*)-1){
perror("shmat failed");
exit(EXIT_FAILURE);
}
//创建信号量
mutex = sem_open(MUTEX_NAME, O_CREAT, 0666, 1);
if(mutex == SEM_FAILED){
perror("sem_open(mutex) failed");
exit(EXIT_FAILURE);
}
full = sem_open(FULL_NAME, O_CREAT, 0666, 0);
if(full == SEM_FAILED){
perror("sem_open(full) failed");
exit(EXIT_FAILURE);
}
printf("请输入一串字符:");
scanf("%s",input);
//获取信号量
P(mutex);
strcpy(shmptr,input);
V(mutex);
V(full);
printf("消息已发送给receiver!\n");
//关闭信号量和共享内存
sem_close(mutex);
sem_close(full);
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <pthread.h> #include <semaphore.h> #include <time.h> #define N 5 pthread_mutex_t chopstick[N];//筷子信号量 //哲学家线程函数 void* philosopher(void* arg){ int *i; i = (int *)arg;//哲学家序号 for(;;){ //思考 printf("%d 号哲学家在思考......\n",*i); sleep(rand()%3);//休眠随机时间,不超过3秒 //尝试取回左右两边的筷子 if(pthread_mutex_trylock(&chopstick[*i]) == 0 && pthread_mutex_trylock(&chopstick[(*i+1)%N]) == 0)//尝试同时拿到两个筷子 { //成功拿到两个筷子 printf("%d号哲学家在进餐......\n",*i); sleep(rand()%3);//休眠随机时间,不超过3秒 //放回筷子 pthread_mutex_unlock(&chopstick[*i]); pthread_mutex_unlock(&chopstick[(*i+1)%N]); } else { //释放已拿到的筷子,等待随机时间后重试 pthread_mutex_unlock(&chopstick[*i]); pthread_mutex_unlock(&chopstick[(*i+1)%N]); sleep(rand()%2); } } } int main(){ pthread_t id[N]; int i; for(i=0;i<N;i++) pthread_mutex_init(&chopstick[i],NULL); for(i=0;i<N;i++) { int *p; p=malloc(sizeof(int)); *p=i; pthread_create(&id[i],NULL,philosopher,(void*)p); } for(i=0;i<N;i++) pthread_join(id[i],NULL); }给代码加注释
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <semaphore.h>
#include <time.h>
#define N 5 //哲学家数量
pthread_mutex_t chopstick[N]; //筷子信号量
//哲学家线程函数
void* philosopher(void* arg){
int *i;
i = (int *)arg; //哲学家序号
for(;;){
//思考
printf("%d 号哲学家在思考......\n",*i);
sleep(rand()%3); //休眠随机时间,不超过3秒
//尝试取回左右两边的筷子
if(pthread_mutex_trylock(&chopstick[*i]) == 0 && pthread_mutex_trylock(&chopstick[(*i+1)%N]) == 0) //尝试同时拿到两个筷子
{
//成功拿到两个筷子
printf("%d号哲学家在进餐......\n",*i);
sleep(rand()%3); //休眠随机时间,不超过3秒
//放回筷子
pthread_mutex_unlock(&chopstick[*i]);
pthread_mutex_unlock(&chopstick[(*i+1)%N]);
}
else
{
//释放已拿到的筷子,等待随机时间后重试
pthread_mutex_unlock(&chopstick[*i]);
pthread_mutex_unlock(&chopstick[(*i+1)%N]);
sleep(rand()%2);
}
}
}
int main(){
pthread_t id[N];
int i;
//初始化筷子信号量
for(i=0;i<N;i++)
pthread_mutex_init(&chopstick[i],NULL);
//创建哲学家线程
for(i=0;i<N;i++)
{
int *p;
p=malloc(sizeof(int));
*p=i;
pthread_create(&id[i],NULL,philosopher,(void*)p);
}
//等待哲学家线程结束
for(i=0;i<N;i++)
pthread_join(id[i],NULL);
}
```
代码中实现了哲学家问题的解决方案,通过线程模拟哲学家的行为,使用互斥锁来解决筷子竞争的问题。其中,`pthread_mutex_init`用于初始化互斥锁,`pthread_create`用于创建线程,`pthread_join`用于等待线程结束。对于每一个哲学家线程,其会进入一个无限循环,先进行思考,然后尝试取回左右两边的筷子,如果成功则进餐,否则释放已拿到的筷子并等待随机时间后重试。
阅读全文
相关推荐
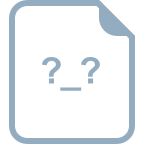
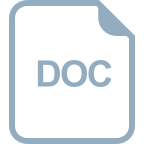
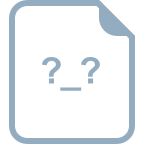





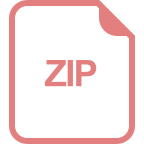