根据提示,在右侧编辑器补充代码,设计一个按字节编址的有32位地址输入端、1位的片选输入端cs和32位数据输出端的只读存储器ROM,其中从地址0开始存放的17条MIPS指令如下表所示。 MIPS指令(16进制) 3c010000 34240050 20050004 0c000018 ac820000 8c890000 01244022 20050003 20a5ffff 34a8ffff 39085555 2009ffff 312affff 01493025 01494026 01463824 10a00001
时间: 2023-07-14 21:12:00 浏览: 102
根据题目要求,需要设计一个按字节编址的ROM,那么我们需要先定义ROM的地址总线、数据总线和片选信号:
```verilog
module ROM(
input [31:0] addr,
input cs,
output reg [31:0] data
);
```
接下来,我们需要将MIPS指令存储到ROM中,并且按字节编址,因此需要将每条指令按照字节存储到ROM中。我们可以定义一个数组来存储这些指令:
```verilog
reg [31:0] instruction[16];
```
然后,我们需要将每一条指令从16进制转换为二进制,并将其存储到instruction数组中:
```verilog
initial begin
instruction[0] = 32'h3c010000;
instruction[1] = 32'h34240050;
instruction[2] = 32'h20050004;
instruction[3] = 32'h0c000018;
instruction[4] = 32'hac820000;
instruction[5] = 32'h8c890000;
instruction[6] = 32'h01244022;
instruction[7] = 32'h20050003;
instruction[8] = 32'h20a5ffff;
instruction[9] = 32'h34a8ffff;
instruction[10] = 32'h39085555;
instruction[11] = 32'h2009ffff;
instruction[12] = 32'h312affff;
instruction[13] = 32'h01493025;
instruction[14] = 32'h01494026;
instruction[15] = 32'h01463824;
instruction[16] = 32'h10a00001;
end
```
最后,我们需要在ROM模块中实现按字节编址的存储器。我们可以使用addr来确定要读取的指令的地址,然后将该地址除以4,得到要读取的指令在instruction数组中的下标。然后,我们需要根据addr的最低两位来确定要读取的字节,然后将该字节从对应的指令中提取出来,作为ROM的输出:
```verilog
always @(addr or cs) begin
if (cs == 1'b0) begin
data <= 32'h0; // 如果片选信号未使能,则输出0
end else begin
case (addr[1:0])
2'b00: data <= instruction[addr[31:2]][7:0];
2'b01: data <= instruction[addr[31:2]][15:8];
2'b10: data <= instruction[addr[31:2]][23:16];
2'b11: data <= instruction[addr[31:2]][31:24];
endcase
end
end
```
完整代码如下:
```verilog
module ROM(
input [31:0] addr,
input cs,
output reg [31:0] data
);
reg [31:0] instruction[16];
initial begin
instruction[0] = 32'h3c010000;
instruction[1] = 32'h34240050;
instruction[2] = 32'h20050004;
instruction[3] = 32'h0c000018;
instruction[4] = 32'hac820000;
instruction[5] = 32'h8c890000;
instruction[6] = 32'h01244022;
instruction[7] = 32'h20050003;
instruction[8] = 32'h20a5ffff;
instruction[9] = 32'h34a8ffff;
instruction[10] = 32'h39085555;
instruction[11] = 32'h2009ffff;
instruction[12] = 32'h312affff;
instruction[13] = 32'h01493025;
instruction[14] = 32'h01494026;
instruction[15] = 32'h01463824;
instruction[16] = 32'h10a00001;
end
always @(addr or cs) begin
if (cs == 1'b0) begin
data <= 32'h0;
end else begin
case (addr[1:0])
2'b00: data <= instruction[addr[31:2]][7:0];
2'b01: data <= instruction[addr[31:2]][15:8];
2'b10: data <= instruction[addr[31:2]][23:16];
2'b11: data <= instruction[addr[31:2]][31:24];
endcase
end
end
endmodule
```
相关推荐
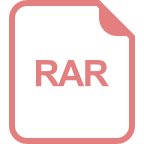
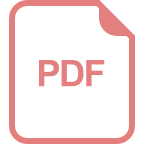














