JAVA如何在子类中打印出父类的所有属性
时间: 2024-09-28 12:11:46 浏览: 27
在Java中,如果想让子类能够访问并打印父类的所有属性,通常需要通过以下步骤:
1. **继承**:首先创建一个父类(Parent),在这个类中定义公共或受保护的属性。
```java
public class Parent {
private String parentAttribute; // 父类属性
public Parent(String attribute) {
this.parentAttribute = attribute;
}
// getter和setter方法
public String getParentAttribute() {
return parentAttribute;
}
public void setParentAttribute(String parentAttribute) {
this.parentAttribute = parentAttribute;
}
}
```
2. **子类**:然后创建一个子类(Child)继承自父类,并可以选择性地覆盖父类的方法或添加新的属性。
```java
public class Child extends Parent {
private String childAttribute;
public Child(String parentAttr, String childAttr) {
super(parentAttr); // 调用父类构造函数
this.childAttribute = childAttr;
}
// 子类可以有自己的getter和setter方法
public String getChildAttribute() {
return childAttribute;
}
public void setChildAttribute(String childAttribute) {
this.childAttribute = childAttribute;
}
@Override
public void printAttributes() { // 重写父类方法
System.out.println("父类属性: " + getParentAttribute());
System.out.println("子类属性: " + getChildAttribute());
}
}
```
3. **使用**:创建子类实例并调用`printAttributes()`方法,会同时打印父类和子类的属性。
```java
Child child = new Child("Parent Attribute", "Child Attribute");
child.printAttributes();
```
阅读全文
相关推荐
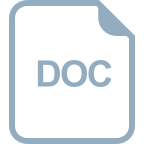
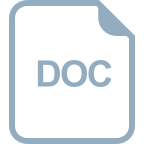
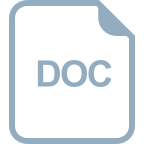

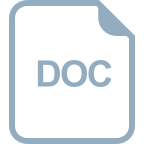
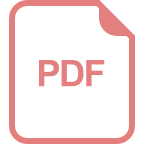
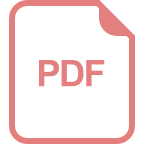












