1. 写一个按钮绑定JavaScript事件用来管理小凳的开关及颜色 按下开关 小灯红色 再次按下 小灯绿色 再次按下 小灯蓝色 再次按下 小灯关闭 2.尝试两种方法(class与style)完成此案例
时间: 2024-09-11 21:09:05 浏览: 32
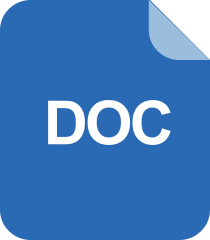
七年级数学下册 第七单元习题 北师大版 试题.doc
1. 首先,创建一个按钮和一个小灯的HTML元素。然后,使用JavaScript为按钮添加点击事件监听器,通过切换类(class)或样式(style)属性来改变小灯的颜色以及开启和关闭小灯。
以下是使用class来完成这个案例的代码示例:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>灯开关示例</title>
<style>
.light {
width: 50px;
height: 50px;
background-color: grey;
border: 1px solid black;
}
.red {
background-color: red;
}
.green {
background-color: green;
}
.blue {
background-color: blue;
}
</style>
<script>
function toggleLight() {
var light = document.getElementById('light');
var state = light.getAttribute('data-state');
if (state === 'off') {
light.setAttribute('data-state', 'red');
light.className = 'light red';
} else if (state === 'red') {
light.setAttribute('data-state', 'green');
light.className = 'light green';
} else if (state === 'green') {
light.setAttribute('data-state', 'blue');
light.className = 'light blue';
} else if (state === 'blue') {
light.setAttribute('data-state', 'off');
light.className = 'light';
}
}
</script>
</head>
<body>
<button onclick="toggleLight()">开关</button>
<div id="light" class="light" data-state="off"></div>
</body>
</html>
```
2. 现在我们尝试使用style属性来完成同样的案例。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>灯开关示例</title>
<script>
function toggleLight() {
var light = document.getElementById('light');
var state = light.getAttribute('data-state');
if (state === 'off') {
light.setAttribute('data-state', 'red');
light.style.backgroundColor = 'red';
} else if (state === 'red') {
light.setAttribute('data-state', 'green');
light.style.backgroundColor = 'green';
} else if (state === 'green') {
light.setAttribute('data-state', 'blue');
light.style.backgroundColor = 'blue';
} else if (state === 'blue') {
light.setAttribute('data-state', 'off');
light.style.backgroundColor = 'grey';
}
}
</script>
</head>
<body>
<button onclick="toggleLight()">开关</button>
<div id="light" style="width: 50px; height: 50px; background-color: grey; border: 1px solid black;" data-state="off"></div>
</body>
</html>
```
在这两个示例中,按钮的点击事件触发了`toggleLight`函数,该函数根据当前小灯的状态(存储在`data-state`属性中)来切换小灯的颜色。当小灯关闭时,它的背景颜色被设置为灰色。
阅读全文
相关推荐
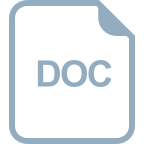
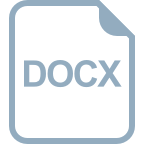
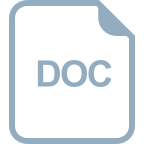
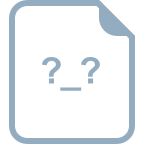
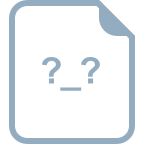
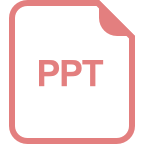
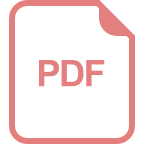
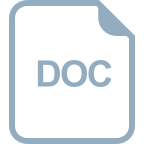
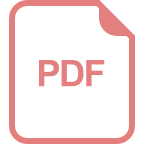
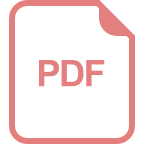
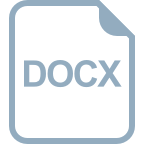
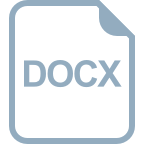
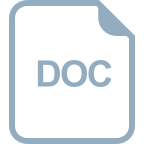


