判断字符串是否为子串c++
时间: 2023-11-11 16:59:31 浏览: 268
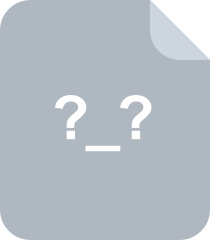
【面向对象的程序设计c++】输入两个字符串,检测第二个字符串是否是第一个字符串的子字符串。
可以使用C++中的string类的find函数来判断一个字符串是否为另一个字符串的子串。如果find函数返回的是string::npos,则说明该字符串不是另一个字符串的子串。
示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "hello world";
string str2 = "world";
if (str1.find(str2) != string::npos) {
cout << str2 << " is a substring of " << str1 << endl;
} else {
cout << str2 << " is not a substring of " << str1 << endl;
}
return 0;
}
```
输出结果为:
```
world is a substring of hello world
```
阅读全文
相关推荐




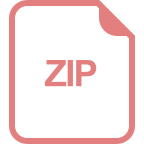
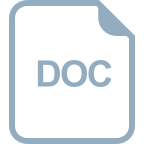









