python模拟退火大规模01规划
时间: 2024-04-04 13:28:26 浏览: 14
Python模拟退火算法是一种常用的优化算法,用于解决大规模01规划问题。模拟退火算法是一种启发式算法,通过模拟金属退火的过程来搜索问题的解空间。
在大规模01规划问题中,我们需要在给定的约束条件下,找到一个二进制向量,使得目标函数取得最大(或最小)值。模拟退火算法通过随机生成初始解,并逐步改进解的质量,最终找到一个接近最优解的解。
以下是使用Python实现模拟退火算法解决大规模01规划问题的步骤:
1. 初始化参数:包括初始温度、终止温度、温度衰减率等。
2. 随机生成初始解:生成一个随机的二进制向量作为初始解。
3. 计算目标函数值:根据问题的目标函数计算当前解的值。
4. 进行状态转移:通过改变当前解的某个元素,生成一个新的解。
5. 计算新解的目标函数值:根据问题的目标函数计算新解的值。
6. 判断是否接受新解:根据一定的概率判断是否接受新解,如果接受,则更新当前解;否则保持当前解不变。
7. 降低温度:根据设定的温度衰减率降低温度。
8. 判断是否达到终止条件:如果温度低于终止温度或达到一定的迭代次数,则停止算法;否则返回步骤4。
通过不断重复上述步骤,模拟退火算法会逐渐收敛到一个较优解。需要注意的是,模拟退火算法是一种启发式算法,不能保证找到全局最优解,但通常能够找到接近最优解的解。
相关问题
模拟退火代码python
模拟退火是一种优化算法,用于在大规模搜索空间中寻找全局最优解。以下是一个简单的模拟退火算法的 Python 代码示例,用于解决 TSP 问题:
```python
import random
import math
# 计算欧几里得距离
def euclidean_distance(point1, point2):
x1, y1 = point1
x2, y2 = point2
return math.sqrt((x1 -
python 退火算法
Python中的退火算法是一种全局优化算法,用于在大规模搜索空间中找到一个接近全局最优解的解。它模拟了金属退火过程中的原理,通过随机搜索和概率接受较差解的方式,在搜索空间中逐渐找到更优的解。
以下是一个简单的示例代码来实现退火算法:
```python
import random
import math
def cost_function(solution):
# 计算适应度函数(成本函数)值,根据具体问题而定
pass
def generate_neighbor(solution):
# 生成邻居解,根据具体问题而定
pass
def acceptance_probability(cost, new_cost, temperature):
if new_cost < cost:
return 1
return math.exp((cost - new_cost) / temperature)
def simulated_annealing(initial_solution, initial_temperature, final_temperature, cooling_rate):
current_solution = initial_solution
best_solution = initial_solution
current_temperature = initial_temperature
while current_temperature > final_temperature:
new_solution = generate_neighbor(current_solution)
current_cost = cost_function(current_solution)
new_cost = cost_function(new_solution)
if acceptance_probability(current_cost, new_cost, current_temperature) > random.random():
current_solution = new_solution
if cost_function(current_solution) < cost_function(best_solution):
best_solution = current_solution
current_temperature *= cooling_rate
return best_solution
# 使用示例
initial_solution = ... # 初始解,据具体问题而定
initial_temperature = ... # 初始温度
final_temperature = ... # 最终温度
cooling_rate = ... # 降温速率
best_solution = simulated_annealing(initial_solution, initial_temperature, final_temperature, cooling_rate)
print(best_solution)
```
其中,`cost_function`函数用于计算解的成本(适应度)函数值,`generate_neighbor`函数用于生成邻居解,`acceptance_probability`函数用于计算接受新解的概率。
在退火算法中,初始温度和降温速率的选择对算法的性能有一定的影响。通常情况下,初始温度应该足够高,以便在搜索空间中进行充分的探索,而降温速率应该足够慢,以充分利用退火的过程。
请注意,以上代码只是一个简单示例,实际问题中需要根据具体情况进行相应的修改和调整。
相关推荐
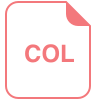
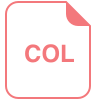
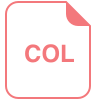
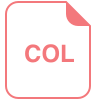
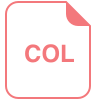







