Python实战指南:模拟退火算法的实现与实践
发布时间: 2024-08-24 20:51:12 阅读量: 36 订阅数: 23 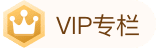
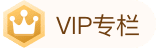
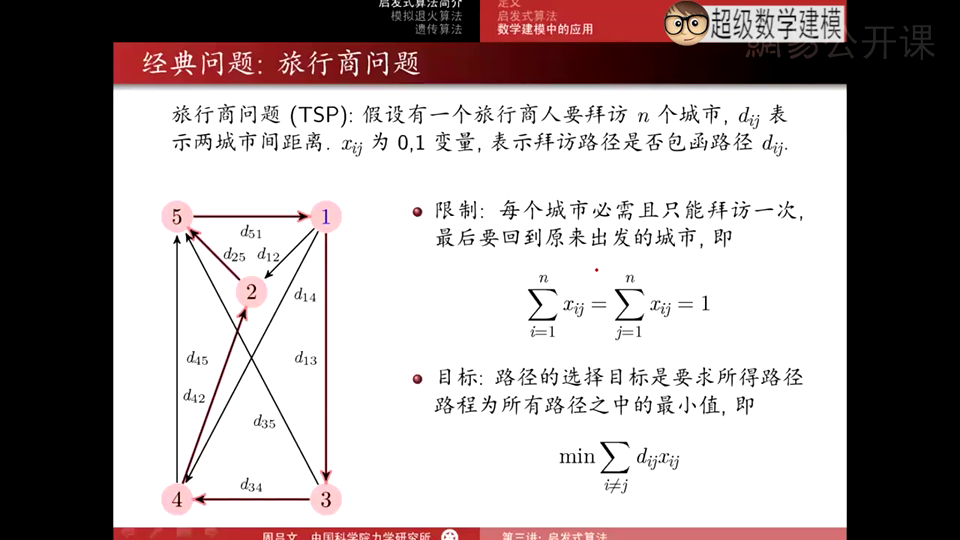
# 1. 模拟退火算法概述**
模拟退火算法是一种受热力学退火过程启发的元启发式优化算法。它通过模拟金属退火过程中的缓慢冷却过程,来寻找给定优化问题的全局最优解。
**基本原理:**
模拟退火算法通过以下步骤进行:
1. 初始化一个随机解。
2. 在当前解的邻域中生成一个新解。
3. 计算新解和当前解的目标函数值之间的差值。
4. 如果新解的目标函数值更优,则接受新解。
5. 否则,以一定概率接受新解。
6. 随着算法的进行,逐渐降低接受新解的概率,直至达到停止条件。
# 2. Python中模拟退火算法的实现
模拟退火算法是一种基于物理退火过程的优化算法,它通过模拟金属退火过程中的能量状态变化,逐步逼近最优解。在Python中,我们可以使用`scipy.optimize`库中的`anneal`函数来实现模拟退火算法。
### Python中实现模拟退火算法的步骤
#### 1. 问题建模和目标函数定义
首先,我们需要将优化问题建模为一个目标函数,该函数将候选解映射到一个数值,表示该解的质量。例如,对于旅行商问题,目标函数可以是旅行总距离。
#### 2. 状态空间和邻域生成
接下来,我们需要定义状态空间,即所有候选解的集合,以及邻域生成函数,该函数将一个状态映射到其相邻的状态集合。对于旅行商问题,状态空间可以是所有可能的旅行路线,而邻域生成函数可以是随机交换两个城市顺序的函数。
#### 3. 退火调度函数设计
最后,我们需要设计一个退火调度函数,该函数控制算法在搜索过程中温度的变化。退火调度函数通常是一个单调递减的函数,随着算法的进行,温度逐渐降低。
### Python代码示例
以下是一个Python代码示例,演示如何使用`scipy.optimize`库中的`anneal`函数实现模拟退火算法:
```python
import numpy as np
from scipy.optimize import anneal
# 目标函数(旅行商问题)
def tsp_cost(route):
# 计算旅行总距离
cost = 0
for i in range(len(route) - 1):
cost += distance_matrix[route[i]][route[i + 1]]
return cost
# 退火调度函数
def tsp_schedule(temperature):
# 随着温度降低,接受率也降低
return temperature / 100
# 邻域生成函数(随机交换两个城市顺序)
def tsp_neighbor(route):
# 随机选择两个城市
i, j = np.random.randint(0, len(route), size=2)
# 交换两个城市顺序
route[i], route[j] = route[j], route[i]
return route
# 初始化参数
initial_state = np.random.permutation(len(cities)) # 随机初始解
max_steps = 10000 # 最大迭代次数
max_temp = 100 # 初始温度
min_temp = 1 # 最低温度
# 使用anneal函数进行模拟退火
result = anneal(tsp_cost, initial_state, schedule=tsp_schedule,
maxiter=max_steps, maxtemp=max_temp, mintemp=min_temp,
neighbor=tsp_neighbor)
# 输出最优解
print("最优解:", result.x)
print("最优成本:", result.fun)
```
**代码逻辑分析:**
* `tsp_cost`函数计算旅行商问题的目标函数,即旅行总距离。
* `tsp_schedule`函数定义了退火调度函数,随着温度降低,接受率也降低。
* `tsp_neighbor`函数定义了邻域生成函数,随机交换两个城市顺序。
* `anneal`函数执行模拟退火算法,并返回最优解和最优成本。
**参数说明:**
* `tsp_cost`:目标函数
* `initial_state`:初始解
* `schedule`:退火调度函数
* `maxiter`:最大迭代次数
* `maxtemp`:初始温度
* `mintemp`:最低温度
* `neighbor`:邻域生成函数
# 3. 模拟退火算法在优化问题中的应用
模拟退火算法是一种强大的优化算法,广泛应用于解决各种优化问题。在本节中,我们将探讨模拟退火算法在两个经典优化问题中的应用:旅行商问题和背包问题。
#### 3.1 旅行商问题
旅行商问题是一个经典的组合优化问题,其目标是找到一条最短的路径,访问给定城市集合中的所有城市一次并返回起点。
**3.1.1 问题描述和数学模型**
旅行商问题可以用数学模型表示为:
```
min f(x) = ∑_{i=1}^{n} ∑_{j=1}^{n} c_{ij} x_{ij}
```
其中:
* f(x) 是目标函数,表示路径的总长度
* c_{ij} 是城市 i 到城市 j 的距离
* x_{ij} 是一个二进制变量,当且仅当路径包含从城市 i 到城市 j 的边时取值为 1
**3.1.2 模拟退火算法求解旅行商问题**
模拟退火算法求解旅行商问题的主要步骤如下:
1. **问题建模:**将问题转换为一个优化问题,定义目标函数和状态空间。
2. **状态空间和邻域生成:**定义状态空间为所有可能的旅行路径,邻域为当前状态的相邻路径。
3. **退火调度函数设计:**设计退火调度函数,以控制算法的退火过程。
4. **算法执行:**从一个初始状态开始,根据退火调度函数逐渐降低温度,并不断更新当前状态,直至达到终止条件。
**代码示例:**
```python
import random
import math
def simulated_annealing(cities, distance_matrix, max_iterations, cooling_rate):
# 初始化
current_state = random.sample(cities, len(cities))
best_state = current_state
best_cost = calculate_cost(current_state, distance_matrix)
temperature = 1.0
# 迭代
for i in range(max_iterations):
# 生成邻域状态
neighbor_state = generate_neighbor(current_state)
# 计算邻域状态的代价
neighbor_cost = calculate_cost(neighbor_state, distance_matrix)
# 计算接受概率
delta_cost = neighbor_cost - current_cost
acceptance_probability = math.exp(-delta_cost / temperature)
# 接受或拒绝邻域状态
if acceptance_probability > random.random():
current_state = neighbor_state
current_cost = neighbor_cost
# 更新最佳状态
if current_cost < best_cost:
best_state = current_state
best_cost = current_cost
# 降低温度
temperature *= cooling_rate
return best_state, best_cost
# 计算路径的总长度
def calculate_cost(state, distance_matrix):
cost = 0
for i in range(len(state) - 1):
cost += distance_matrix[state[i]][state[i+1]]
```
0
0
相关推荐
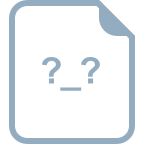
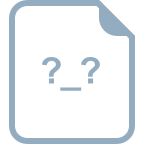
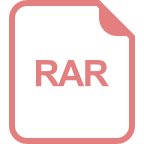





