优化问题秒变简单!模拟退火算法的实战案例解析
发布时间: 2024-08-24 20:46:11 阅读量: 61 订阅数: 24 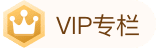
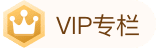
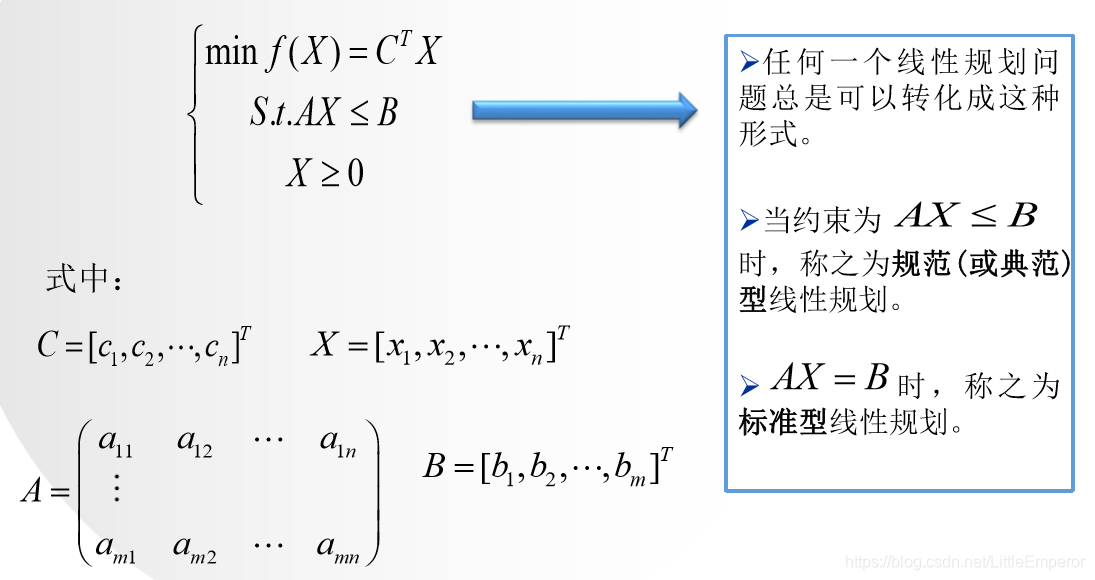
# 1. 模拟退火算法概述**
模拟退火算法是一种基于概率的优化算法,灵感来源于物理学中固体退火过程。它通过模拟固体从高温逐渐冷却到低温的过程,在搜索空间中寻找最优解。
该算法的核心思想是:在搜索过程中,允许一定程度的随机扰动,以避免陷入局部最优解。随着算法的进行,扰动的幅度逐渐减小,最终收敛到全局最优解附近。
# 2. 模拟退火算法理论基础
### 2.1 概率分布与马尔可夫链
**2.1.1 概率分布**
概率分布描述了随机变量可能取值的概率。在模拟退火算法中,我们使用概率分布来指导解空间的探索。
**2.1.2 马尔可夫链**
马尔可夫链是一种随机过程,其中每个状态的未来演变只取决于当前状态,与过去状态无关。在模拟退火算法中,我们使用马尔可夫链来模拟解空间的移动。
### 2.2 模拟退火算法的基本原理
模拟退火算法是一种基于概率的优化算法,它模拟了金属退火的过程。金属退火通过缓慢降低温度来使金属达到更稳定的状态。
**2.2.1 基本流程**
模拟退火算法的基本流程如下:
1. **初始化:**初始化解和温度。
2. **生成邻域解:**根据当前解,生成一个邻域解。
3. **计算能量差:**计算当前解和邻域解之间的能量差。
4. **接受或拒绝:**如果能量差为负,则接受邻域解;否则,根据概率接受或拒绝邻域解。
5. **更新温度:**根据退火策略更新温度。
6. **重复步骤 2-5:**重复上述步骤,直到达到停止条件。
**2.2.2 概率接受准则**
在模拟退火算法中,我们使用概率接受准则来决定是否接受邻域解。概率接受准则为:
```
P(accept) = exp(-ΔE / T)
```
其中:
* ΔE 为能量差
* T 为温度
**2.2.3 退火策略**
退火策略决定了温度如何随时间变化。常见的退火策略包括:
* **线性退火:**温度以恒定的速率降低。
* **指数退火:**温度以指数速率降低。
* **自适应退火:**温度根据算法的性能进行调整。
### 代码示例
以下代码展示了模拟退火算法的基本实现:
```python
import random
def simulated_annealing(initial_solution, temperature, cooling_rate):
current_solution = initial_solution
best_solution = initial_solution
while temperature > 0:
# Generate a neighbor solution
neighbor_solution = generate_neighbor(current_solution)
# Calculate the energy difference
energy_diff = calculate_energy_diff(current_solution, neighbor_solution)
# Accept or reject the neighbor solution
if energy_diff < 0 or random.random() < math.exp(-energy_diff / temperature):
current_solution = neighbor_solution
# Update the best solution
if calculate_energy(current_solution) < calculate_energy(best_solution):
best_solution = current_solution
# Update the temperature
temperature *= cooling_rate
return best_solution
```
**代码逻辑分析:**
* 函数 `simulated_annealing` 接受初始解、温度和冷却速率作为参数。
* 初始化当前解和最佳解为初始解。
* 循环执行以下步骤,直到温度为 0:
* 生成一个邻域解。
* 计算当前解和邻域解之间的能量差。
* 根据概率接受准则接受或拒绝邻域解。
* 如果邻域解被接受,则更新当前解。
* 如果当前解的能量比最佳解的能量低,则更新最佳解。
* 更新温度。
* 返回最佳解。
# 3. 模拟退火算法实践应用**
**3.1 旅行商问题求解**
旅行商问题(TSP)是一个经典的组合优化问题,目标是找到一条最短的路径,使得该路径经过给定的城市集合一次且仅一次。模拟退火算法可以有效地求解TSP问题。
**3.1.1 问题建模**
将城市集合表示为一个图,其中城市为节点,城市之间的距离为边权重。TSP问题的目标是找到一条哈密顿回路,即一条经过所有城市且不重复的回路。
**3.1.2 模拟退火算法求解**
1. **初始化:**随机生成一个哈密顿回路作为初始解。
2. **扰动:**通过交换两个城市的位置或插入一个城市来扰动当前解。
3. **接受准则:**根据 Metropolis-Hastings 准则接受或拒绝扰动后的解。如果扰动后的解比当前解更好,则直接接受;否则,以一定概率接受。
4. **降温:**随着迭代次数的增加,逐渐降低温度。温度越高,接受较差解的概率越大;温度越低,接受较差解的概率越小。
5. **终止:**当达到最大迭代次数或温度降至足够低时,算法终止。
**代码块:**
```python
import random
import math
def simulated_annealing_tsp(cities, max_iterations, initial_temperature, cooling_rate):
# 初始化
current_solution = random.sample(cities, len(cities))
best_solution = current_solution
best_cost = calculate_cost(current_solution)
# 模拟退火循环
for iteration in range(max_iterations):
# 降温
temperature = initial_temperature * cooling_rate ** iteration
# 扰动
new_solution = perturb(current_solution)
new_cost = calculate_cost(new_solution)
# 接受准则
if new_cost < best_cost or random.random() < math.exp((best_cost - new_cost) / temperature):
current_solution = new_solution
if new_cost < best_cost:
best_solution = new_solution
best_cost = new_cost
return best_solution
# 计算路径成本
def calculate_cost(solution):
cost = 0
for i in range(len(solution)):
cost += distance_matrix[solution[i]][solution[(i + 1) % len(solution)]]
return cost
# 扰动路径
def perturb(solution):
i, j = random.sample(range(len(solution)), 2)
new_solution = solution.copy()
new_solution[i], new_solution[j] = new_solution[j], new_solution[i]
return new_solution
```
**逻辑分析:**
* 初始化阶段:随机生成一个哈密顿回路作为初始解,并将其作为当前解和最佳解。
* 扰动阶段:通过交换两个城市的位置或插入一个城市来扰动当前解,产生一个新的解。
* 接受准则:根据 Metropolis-Hastings 准则接受或拒绝扰动后的解。如果扰动后的解比当前解更好,则直接接受;否则,以一定概率接受。
* 降温阶段:随着迭代次数的增加,逐渐降低温度。温度越高,接受较差解的概率越大;温度越低,接受较差解的概率越小。
* 终止阶段:当达到最大迭代次数或温度降至足够低时,算法终止,并返回最佳解。
**3.2 图像分割优化**
图像分割是将图像分割成具有不同特征的区域的过程。模拟退火算法可以用于优化图像分割结果。
**3.2.1 问题建模**
将图像表示为一个像素集合,每个像素都有一个颜色值。图像分割的目标是将像素分配到不同的区域,使得每个区域内的像素颜色值相似。
**3.2.2 模拟退火算法求解**
1. **初始化:**随机初始化一个分割方案,即每个像素分配到一个区域。
2. **扰动:**通过改变一个像素的区域分配来扰动当前分割方案。
3. **接受准则:**根据 Metropolis-Hastings 准则接受或拒绝扰动后的分割方案。如果扰动后的分割方案比当前分割方案更好,则直接接受;否则,以一定概率接受。
4. **降温:**随着迭代次数的增加,逐渐降低温度。温度越高,接受较差分割方案的概率越大;温度越低,接受较差分割方案的概率越小。
5. **终止:**当达到最大迭代次数或温度降至足够低时,算法终止。
**代码块:**
```python
import numpy as np
import cv2
def simulated_annealing_image_segmentation(image, max_iterations, initial_temperature, cooling_rate):
# 初始化
current_segmentation = np.random.randint(0, 256, size=image.shape[:2])
best_segmentation = current_segmentation
best_cost = calculate_cost(image, current_segmentation)
# 模拟退火循环
for iteration in range(max_iterations):
# 降温
temperature = initial_temperature * cooling_rate ** iteration
# 扰动
new_segmentation = perturb(current_segmentation)
new_cost = calculate_cost(image, new_segmentation)
# 接受准则
if new_cost < best_cost or random.random() < math.exp((best_cost - new_cost) / temperature):
current_segmentation = new_segmentation
if new_cost < best_cost:
best_segmentation = new_segmentation
best_cost = new_cost
return best_segmentation
# 计算分割成本
def calculate_cost(image, segmentation):
cost = 0
for i in range(image.shape[0]):
for j in range(image.shape[1]):
cost += (image[i, j] - image[i, j][segmentation[i, j]]) ** 2
return cost
# 扰动分割
def perturb(segmentation):
i, j = random.sample(range(segmentation.shape[0]), 2)
new_segmentation = segmentation.copy()
new_segmentation[i, j] = random.randint(0, 255)
return new_segmentation
```
**逻辑分析:**
* 初始化阶段:随机初始化一个分割方案,即每个像素分配到一个区域。
* 扰动阶段:通过改变一个像素的区域分配来扰动当前分割方案,产生一个新的分割方案。
* 接受准则:根据 Metropolis-Hastings 准则接受或拒绝扰动后的分割方案。如果扰动后的分割方案比当前分割方案更好,则直接接受;否则,以一定概率接受。
* 降温阶段:随着迭代次数的增加,逐渐降低温度。温度越高,接受较差分割方案的概率越大;温度越低,接受较差分割方案的概率越小。
* 终止阶段:当达到最大迭代次数或温度降至足够低时,算法终止,并返回最佳分割方案。
# 4. 模拟退火算法进阶技巧
### 4.1 退火策略与参数选择
**退火策略**
退火策略决定了算法降温的速率,影响算法的收敛速度和解的质量。常见的退火策略有:
* **线性退火:**温度按线性速率下降,即 `T(k) = T(0) * (1 - k / K)`,其中 `T(0)` 为初始温度,`k` 为当前迭代次数,`K` 为最大迭代次数。
* **指数退火:**温度按指数速率下降,即 `T(k) = T(0) * exp(-k / K)`。
* **对数退火:**温度按对数速率下降,即 `T(k) = T(0) / log(k + 1)`。
**参数选择**
模拟退火算法的参数选择对算法性能至关重要。主要参数包括:
* **初始温度:**初始温度过高,算法可能跳出最优解区域;过低,算法可能陷入局部最优解。
* **降温速率:**降温速率过快,算法可能无法充分探索搜索空间;过慢,算法收敛速度慢。
* **迭代次数:**迭代次数过少,算法可能无法找到最优解;过多,算法计算量大。
### 4.2 算法并行化与分布式实现
**并行化**
模拟退火算法可以并行化,以提高计算效率。并行化方法包括:
* **多线程并行:**将算法拆分为多个线程,同时执行。
* **GPU 并行:**利用 GPU 的并行计算能力,加速算法执行。
**分布式实现**
对于大规模问题,模拟退火算法还可以分布式实现,将算法任务分配到多个计算节点上执行。分布式实现方法包括:
* **消息传递接口(MPI):**使用 MPI 库实现进程间通信,协调算法执行。
* **Hadoop:**使用 Hadoop 分布式计算框架,将算法任务分配到 Hadoop 集群上执行。
**代码示例**
```python
import numpy as np
import random
# 模拟退火算法
class SimulatedAnnealing:
def __init__(self, problem, initial_temperature, cooling_rate, max_iterations):
self.problem = problem
self.initial_temperature = initial_temperature
self.cooling_rate = cooling_rate
self.max_iterations = max_iterations
def solve(self):
# 初始化
current_solution = self.problem.generate_random_solution()
current_cost = self.problem.evaluate(current_solution)
best_solution = current_solution
best_cost = current_cost
temperature = self.initial_temperature
# 迭代
for i in range(self.max_iterations):
# 生成邻域解
neighbor_solution = self.problem.generate_neighbor_solution(current_solution)
neighbor_cost = self.problem.evaluate(neighbor_solution)
# 计算接受概率
delta_cost = neighbor_cost - current_cost
if delta_cost < 0:
probability = 1.0
else:
probability = np.exp(-delta_cost / temperature)
# 接受或拒绝邻域解
if random.random() < probability:
current_solution = neighbor_solution
current_cost = neighbor_cost
# 更新最优解
if current_cost < best_cost:
best_solution = current_solution
best_cost = current_cost
# 降温
temperature *= self.cooling_rate
return best_solution, best_cost
```
**代码逻辑分析**
* 初始化算法参数,包括问题对象、初始温度、降温速率和最大迭代次数。
* 初始化当前解、当前解的代价、最优解和最优解的代价。
* 迭代算法,每次迭代生成邻域解,计算接受概率,接受或拒绝邻域解,更新最优解,并降温。
* 返回最优解和最优解的代价。
# 5.1 物流配送优化
模拟退火算法在物流配送优化中有着广泛的应用,其目标是设计一条最优配送路线,以最小化配送成本或时间。
**问题描述:**
给定一个城市列表及其之间的距离,以及每个城市需要配送的货物数量。目标是找到一条配送路线,满足以下条件:
* 访问所有城市一次且仅一次
* 总配送距离或时间最小
**模拟退火算法应用:**
1. **初始化:**生成一个随机配送路线,并计算其总配送距离或时间。
2. **扰动:**随机选择两个城市,交换它们的顺序,生成一个新的配送路线。
3. **接受准则:**计算新配送路线的总配送距离或时间。如果新配送路线的总配送距离或时间更小,则接受新配送路线。否则,根据概率接受新配送路线。
4. **退火:**随着迭代次数的增加,逐渐降低接受新配送路线的概率。
5. **循环:**重复步骤 2-4,直到达到停止条件(例如,达到最大迭代次数或总配送距离或时间不再改善)。
**代码示例:**
```python
import random
def simulated_annealing(cities, distances, num_iterations):
# 初始化
current_route = random.sample(cities, len(cities))
current_cost = calculate_total_distance(current_route, distances)
best_route = current_route
best_cost = current_cost
# 循环
for i in range(num_iterations):
# 扰动
new_route = current_route[:]
a, b = random.sample(range(len(cities)), 2)
new_route[a], new_route[b] = new_route[b], new_route[a]
# 计算新配送路线的总配送距离或时间
new_cost = calculate_total_distance(new_route, distances)
# 接受准则
if new_cost < current_cost or random.random() < acceptance_probability(current_cost, new_cost, i, num_iterations):
current_route = new_route
current_cost = new_cost
# 更新最佳配送路线
if current_cost < best_cost:
best_route = current_route
best_cost = current_cost
return best_route
def calculate_total_distance(route, distances):
total_distance = 0
for i in range(len(route) - 1):
total_distance += distances[route[i]][route[i+1]]
return total_distance
def acceptance_probability(current_cost, new_cost, i, num_iterations):
# 退火策略
temperature = num_iterations / (i + 1)
return math.exp(-(new_cost - current_cost) / temperature)
```
**优化技巧:**
* **选择合适的退火策略:**指数退火、线性退火或对数退火。
* **调整退火参数:**初始温度、降温速率和停止条件。
* **并行化算法:**利用多核或分布式计算资源。
0
0
相关推荐
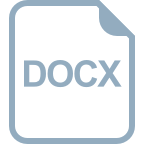
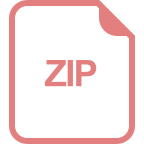
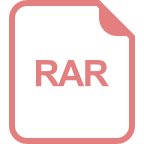
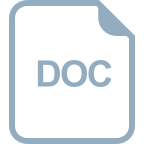
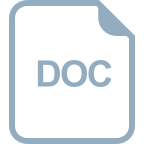
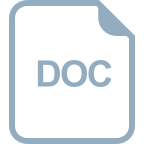
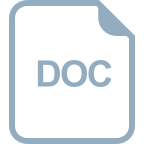
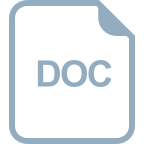
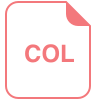