走迷宫问题c语言代码实现
时间: 2024-04-30 08:25:19 浏览: 183
以下是迷宫问题的C语言代码实现。该代码使用了深度优先搜索算法来寻找迷宫的解。
```c
#include <stdio.h>
#define ROW 5
#define COL 5
int maze[ROW][COL] = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0}
};
int solution[ROW][COL] = {0};
int is_valid(int row, int col) {
if (row < 0 || row >= ROW || col < 0 || col >= COL) {
return 0;
}
if (maze[row][col] == 1 || solution[row][col] == 1) {
return 0;
}
return 1;
}
int solve(int row, int col) {
if (row == ROW - 1 && col == COL - 1) {
solution[row][col] = 1;
return 1;
}
if (is_valid(row, col)) {
solution[row][col] = 1;
if (solve(row, col + 1)) {
return 1;
}
if (solve(row + 1, col)) {
return 1;
}
if (solve(row, col - 1)) {
return 1;
}
if (solve(row - 1, col)) {
return 1;
}
solution[row][col] = 0;
}
return 0;
}
int main() {
if (solve(0, 0)) {
printf("Solution found!\n");
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
printf("%d ", solution[i][j]);
}
printf("\n");
}
} else {
printf("Solution not found!\n");
}
return 0;
}
```
该代码中的 `maze` 数组表示迷宫的布局,其中 `0` 表示可通过的路径,`1` 表示障碍物。 `solution` 数组用于保存找到的解。 `is_valid` 函数用于检查给定的位置是否可以通过。 `solve` 函数使用深度优先搜索算法来寻找迷宫的解。 `main` 函数调用 `solve` 函数,并在找到解时打印出 `solution` 数组。
阅读全文
相关推荐

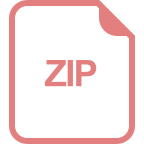
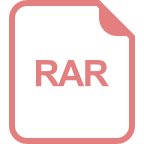
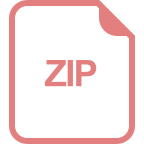
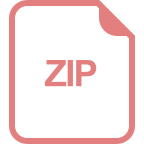
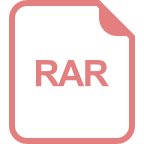







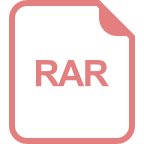