51单片机运用spi协议的sd卡模块
时间: 2023-05-13 13:05:41 浏览: 59
可以使用以下代码来实现:
#include <reg51.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit CS = P1^4; // SD卡片选引脚
sbit MOSI = P1^5; // SD卡数据输出引脚
sbit MISO = P1^6; // SD卡数据输入引脚
sbit SCK = P1^7; // SD卡时钟引脚
void delay(uint i)
{
while(i--);
}
void spi_write(uchar dat)
{
uchar i;
for(i=0; i<8; i++)
{
MOSI = dat & 0x80;
dat <<= 1;
SCK = 1;
_nop_();
_nop_();
SCK = 0;
}
}
uchar spi_read(void)
{
uchar i, dat = 0;
for(i=0; i<8; i++)
{
SCK = 1;
_nop_();
_nop_();
dat <<= 1;
dat |= MISO;
SCK = 0;
}
return dat;
}
uchar sd_init(void)
{
uchar i, r1;
CS = 1;
for(i=0; i<10; i++)
{
spi_write(0xff);
}
CS = 0;
spi_write(0x40);
spi_write(0x00);
spi_write(0x00);
spi_write(0x00);
spi_write(0x00);
spi_write(0x95);
r1 = spi_read();
CS = 1;
spi_write(0xff);
return r1;
}
uchar sd_read_sector(uint sector, uchar *buf)
{
uchar i, r1;
CS = 1;
spi_write(0xff);
CS = 0;
spi_write(0x51);
spi_write((sector >> 24) & 0xff);
spi_write((sector >> 16) & 0xff);
spi_write((sector >> 8) & 0xff);
spi_write(sector & 0xff);
spi_write(0xff);
r1 = spi_read();
if(r1 != 0x00)
{
CS = 1;
spi_write(0xff);
return r1;
}
while(spi_read() != 0xfe);
for(i=0; i<512; i++)
{
buf[i] = spi_read();
}
spi_write(0xff);
spi_write(0xff);
CS = 1;
spi_write(0xff);
return 0;
}
uchar sd_write_sector(uint sector, uchar *buf)
{
uchar i, r1;
CS = 1;
spi_write(0xff);
CS = 0;
spi_write(0x58);
spi_write((sector >> 24) & 0xff);
spi_write((sector >> 16) & 0xff);
spi_write((sector >> 8) & 0xff);
spi_write(sector & 0xff);
spi_write(0xff);
spi_write(0xfe);
for(i=0; i<512; i++)
{
spi_write(buf[i]);
}
spi_write(0xff);
spi_write(0xff);
r1 = spi_read();
if((r1 & 0x1f) != 0x05)
{
CS = 1;
spi_write(0xff);
return r1;
}
while(!spi_read());
CS = 1;
spi_write(0xff);
return 0;
}
int main()
{
uchar buf[512];
uint i;
sd_init();
for(i=0; i<512; i++)
{
buf[i] = i & 0xff;
}
sd_write_sector(0, buf);
sd_read_sector(0, buf);
return 0;
}
关于中文加密,可以使用以下代码来实现:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define uchar unsigned char
#define uint unsigned int
void encrypt(char *str, char *key)
{
uint i, j, k, len1, len2;
len1 = strlen(str);
len2 = strlen(key);
for(i=0, j=0; i<len1; i++, j++)
{
if(j >= len2)
{
j = 0;
}
k = str[i] + key[j];
if(k > 0xff)
{
k -= 0xff;
}
str[i] = k;
}
}
void decrypt(char *str, char *key)
{
uint i, j, k, len1, len2;
len1 = strlen(str);
len2 = strlen(key);
for(i=0, j=0; i<len1; i++, j++)
{
if(j >= len2)
{
j = 0;
}
k = str[i] - key[j];
if(k < 0)
{
k += 0xff;
}
str[i] = k;
}
}
int main()
{
char str[] = "中文加密测试";
char key[] = "123456";
encrypt(str, key);
printf("加密后:%s\n", str);
decrypt(str, key);
printf("解密后:%s\n", str);
return 0;
}
相关推荐
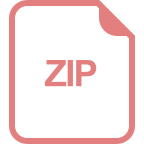
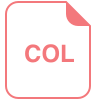
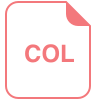
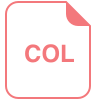
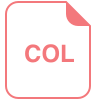
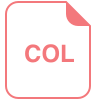









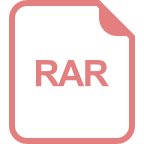