def _inference_viewpoint(self, keypoints_scoremap, hand_side, evaluation, train=False): """ Inference of the viewpoint. """ with tf.variable_scope('ViewpointNet'): # estimate rotation ux, uy, uz = self._rotation_estimation(keypoints_scoremap, hand_side, evaluation, train=train) # assemble rotation matrix rot_mat = self._get_rot_mat(ux, uy, uz) return rot_mat注释
时间: 2024-02-14 14:07:03 浏览: 23
这段代码是一个用于推断手部视角的函数,其输入为关键点的得分图、手的侧面信息以及是否为评估模式。在该函数中,首先使用另一个函数 `_rotation_estimation()` 来估计手的旋转角度,其中 `ux`、`uy`、`uz` 分别表示绕x、y、z轴旋转的角度。然后,使用 `_get_rot_mat()` 函数将旋转角度组装成旋转矩阵 `rot_mat`。最终,返回旋转矩阵 `rot_mat` 作为结果。这个函数的输出可以用于将手的姿态从一个视角转换到另一个视角。
相关问题
def _inference_pose3d_can(self, keypoints_scoremap, hand_side, evaluation, train=False): """ Inference of canonical coordinates. """ with tf.variable_scope('PosePrior'): # use encoding to detect relative, normed 3d coords x = keypoints_scoremap # this is 28x28x21 s = x.get_shape().as_list() out_chan_list = [32, 64, 128] for i, out_chan in enumerate(out_chan_list): x = ops.conv_relu(x, 'conv_pose_%d_1' % i, kernel_size=3, stride=1, out_chan=out_chan, trainable=train) x = ops.conv_relu(x, 'conv_pose_%d_2' % i, kernel_size=3, stride=2, out_chan=out_chan, trainable=train) # in the end this will be 4x4xC注释
这段代码是一个用于推断3D姿态的函数,其输入为关键点的得分图、手的侧面信息以及是否为评估模式。在该函数中,使用编码信息来检测相对的规范化的3D坐标。具体地,首先将关键点得分图经过一系列卷积和ReLU激活函数操作后,得到一个形状为28x28x21的张量x。然后,将该张量x经过三个不同的卷积层,每个卷积层都包含两个卷积操作和ReLU激活函数,最终得到一个形状为4x4xC的张量x,其中C是输出通道数,表示规范化的3D坐标。该函数的输出是规范化的3D坐标。
s = image_crop.get_shape().as_list() keypoints_scoremap = self.inference_pose2d(image_crop) keypoints_scoremap = keypoints_scoremap[-1] keypoints_scoremap = tf.image.resize_images(keypoints_scoremap, (s[1], s[2])) return keypoints_scoremap, image_crop, scale_crop, center注释
这段代码的作用是对输入的图像进行裁剪,并对裁剪后的图像进行姿态估计(pose estimation),得到一个关键点(keypoints)的分数地图(score map)。具体来说,代码的执行过程如下:
1. 获取输入图像的形状(shape)信息,并将其转换为列表形式,保存在变量s中。
2. 对输入图像进行裁剪操作,得到裁剪后的图像,保存在变量image_crop中。
3. 调用self.inference_pose2d()方法,对裁剪后的图像进行姿态估计,得到一个关键点的分数地图,保存在变量keypoints_scoremap中。
4. 由于关键点的分数地图是一个多层的张量(tensor),其中最后一层才是真正的分数地图,因此使用[-1]索引操作,将最后一层分数地图提取出来,并重新赋值给keypoints_scoremap变量。
5. 对提取出来的分数地图进行图像尺寸的调整(resize),使其与输入图像的尺寸相同,保存在变量keypoints_scoremap中。
6. 返回关键点的分数地图、裁剪后的图像、裁剪比例(scale_crop)和图像中心点(center)。
相关推荐
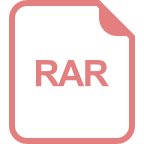
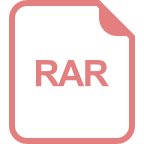












