如何用c加加加密一个文件
时间: 2023-04-08 08:02:41 浏览: 79
可以使用 OpenSSL 库中的加密函数来实现文件加密。具体步骤如下:
1. 打开需要加密的文件和加密后的文件,以二进制方式读写文件。
2. 生成一个随机的密钥,可以使用 OpenSSL 库中的随机数生成函数。
3. 使用 AES 算法加密文件,可以使用 OpenSSL 库中的 EVP 加密函数。需要设置加密模式、密钥、IV 等参数。
4. 将生成的密钥写入加密后的文件头部,以便解密时使用。
5. 关闭文件,释放资源。
需要注意的是,加密后的文件需要保护好密钥,以免泄露导致文件被解密。
相关问题
rsa文件加密c加加代码
以下是使用C语言实现RSA文件加密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#define KEY_LENGTH 2048 // RSA密钥长度
#define BLOCK_SIZE 245 // 加密块大小,需要小于KEY_LENGTH/8-11
int main()
{
RSA *rsa = NULL;
FILE *fp = NULL;
char *pub_key_file = "public_key.pem"; // 公钥文件名
char *in_file = "input.txt"; // 待加密文件名
char *out_file = "output.txt"; // 加密后文件名
// 读取公钥文件
if ((fp = fopen(pub_key_file, "rb")) == NULL)
{
printf("Error: cannot open public key file!\n");
return -1;
}
if ((rsa = PEM_read_RSA_PUBKEY(fp, NULL, NULL, NULL)) == NULL)
{
printf("Error: cannot read public key file!\n");
return -1;
}
fclose(fp);
// 打开待加密文件
if ((fp = fopen(in_file, "rb")) == NULL)
{
printf("Error: cannot open input file!\n");
RSA_free(rsa);
return -1;
}
// 创建加密后文件
FILE *fout = NULL;
if ((fout = fopen(out_file, "wb")) == NULL)
{
printf("Error: cannot create output file!\n");
RSA_free(rsa);
fclose(fp);
return -1;
}
// 分块加密
int block_size = BLOCK_SIZE;
unsigned char *in = (unsigned char *)malloc(block_size);
unsigned char *out = (unsigned char *)malloc(KEY_LENGTH/8);
int in_len, out_len;
while ((in_len = fread(in, 1, block_size, fp)) > 0)
{
out_len = RSA_public_encrypt(in_len, in, out, rsa, RSA_PKCS1_PADDING);
if (out_len == -1)
{
printf("Error: RSA encryption failed!\n");
RSA_free(rsa);
fclose(fp);
fclose(fout);
free(in);
free(out);
return -1;
}
fwrite(out, 1, out_len, fout);
}
// 释放资源
RSA_free(rsa);
fclose(fp);
fclose(fout);
free(in);
free(out);
printf("Encryption successful!\n");
return 0;
}
```
需要注意的是,该代码使用了OpenSSL库来实现RSA加密,因此需要在编译时链接该库。例如,使用gcc编译命令如下:
```
gcc -o rsa_encrypt rsa_encrypt.c -lcrypto
```
其中,"-lcrypto"选项表示链接OpenSSL库。
用C加加写一个读取Excel文件的代码
首先,您需要安装一个用于读取Excel文件的库,比如说EasyXLS或者EPPlus。然后,您可以使用下面的代码来读取Excel文件:
```c++
#include "easyxls.h"
#include <iostream>
int main()
{
// 创建一个Excel文件读取器
xlsEasyXLSReader reader;
// 打开Excel文件
if (reader.easy_LoadXLSFile("example.xls"))
{
// 获取工作表
xlsWorksheet* sheet = reader.easy_getSheet(0);
// 读取工作表中的每一行
for (int rowIndex = 0; rowIndex <= sheet->lastUsedRowIndex(); rowIndex++)
{
xlsRow* row = sheet->getRow(rowIndex);
// 读取每一列
for (int colIndex = 0; colIndex <= row->lastUsedColIndex(); colIndex++)
{
xlsCell* cell = row->getCell(colIndex);
std::cout << cell->getValue() << " ";
}
std::cout << std::endl;
}
// 关闭Excel文件
reader.easy_CloseXLSFile();
}
return 0;
}
```
注意:上面的代码使用的是EasyXLS库,您可以根据所使用的不同的库来更改代码。
阅读全文
相关推荐
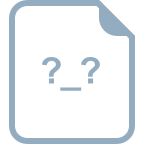













