rsa文件加密c加加代码不用openssl库
时间: 2023-10-12 17:25:36 浏览: 150
RSA加密算法是一种非对称加密算法,使用公钥对数据进行加密,使用私钥对数据进行解密。以下是一个简单的C++代码示例,可以使用RSA算法对文件进行加密,不使用OpenSSL库:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <cstdlib>
#include <ctime>
#include <cmath>
using namespace std;
const int MAX = 1000;
// 欧几里德算法求最大公约数
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
// 扩展欧几里德算法求模反元素
int exgcd(int a, int b, int &x, int &y) {
if (b == 0) {
x = 1;
y = 0;
return a;
}
int d = exgcd(b, a % b, x, y);
int t = x;
x = y;
y = t - a / b * y;
return d;
}
// 生成大质数
int generate_prime(int n) {
srand(time(NULL));
while (true) {
int p = rand() % n + n / 2;
bool flag = true;
for (int i = 2; i <= sqrt(p); i++) {
if (p % i == 0) {
flag = false;
break;
}
}
if (flag) {
return p;
}
}
}
// 生成公钥和私钥
void generate_key(int &p, int &q, int &n, int &e, int &d) {
p = generate_prime(MAX);
q = generate_prime(MAX);
n = p * q;
int f = (p - 1) * (q - 1);
e = 65537;
while (gcd(f, e) != 1) {
e++;
}
exgcd(e, f, d, n);
d = (d % f + f) % f;
}
// RSA加密
void encrypt(const char *input_file, const char *output_file, int n, int e) {
ifstream fin(input_file, ios::binary | ios::in);
ofstream fout(output_file, ios::binary | ios::out);
if (!fin.is_open() || !fout.is_open()) {
return;
}
char buffer[MAX];
while (!fin.eof()) {
fin.read(buffer, MAX);
int len = fin.gcount();
for (int i = 0; i < len; i++) {
int m = buffer[i];
int c = 1;
for (int j = 0; j < e; j++) {
c = (c * m) % n;
}
fout.write((char *)&c, sizeof(int));
}
}
fin.close();
fout.close();
}
// RSA解密
void decrypt(const char *input_file, const char *output_file, int n, int d) {
ifstream fin(input_file, ios::binary | ios::in);
ofstream fout(output_file, ios::binary | ios::out);
if (!fin.is_open() || !fout.is_open()) {
return;
}
int buffer[MAX];
while (!fin.eof()) {
fin.read((char *)buffer, sizeof(int) * MAX);
int len = fin.gcount() / sizeof(int);
for (int i = 0; i < len; i++) {
int c = buffer[i];
int m = 1;
for (int j = 0; j < d; j++) {
m = (m * c) % n;
}
fout.write((char *)&m, sizeof(char));
}
}
fin.close();
fout.close();
}
int main() {
int p, q, n, e, d;
generate_key(p, q, n, e, d);
cout << "p = " << p << endl;
cout << "q = " << q << endl;
cout << "n = " << n << endl;
cout << "e = " << e << endl;
cout << "d = " << d << endl;
encrypt("input.txt", "output.txt", n, e);
decrypt("output.txt", "input2.txt", n, d);
return 0;
}
```
上述代码首先通过欧几里德算法求出最大公约数,再使用扩展欧几里德算法求出模反元素,进而生成公钥和私钥。然后通过文件输入输出流,读取需要加密的文件并加密后输出到输出文件中。最后再通过私钥对输出文件进行解密。
阅读全文
相关推荐








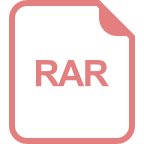


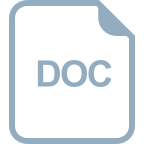





