使用openssl3.0库 实现CRT加速的RSA加密,并和普通的RSA加密比较,代码实现
时间: 2023-06-14 13:06:36 浏览: 181
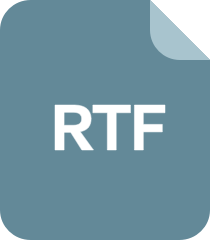
使用openssl实现RSA加解密
使用 OpenSSL 3.0 库实现 CRT 加速的 RSA 加密可以通过以下步骤实现:
1. 初始化 OpenSSL 库和随机数生成器:
```c
#include <openssl/rand.h>
#include <openssl/ssl.h>
#include <openssl/evp.h>
// 初始化 OpenSSL 库和随机数生成器
void init_openssl() {
SSL_library_init();
SSL_load_error_strings();
ERR_load_crypto_strings();
OpenSSL_add_all_algorithms();
// 随机数生成器需要初始化
RAND_poll();
}
```
2. 生成 RSA 密钥对:
```c
#include <openssl/rsa.h>
#include <openssl/bn.h>
// 生成 RSA 密钥对
RSA* generate_rsa_key_pair(int bits) {
RSA* rsa = RSA_new();
BIGNUM* bne = BN_new();
// 生成指数
if (BN_set_word(bne, RSA_F4) != 1) {
printf("Failed to set RSA exponent.\n");
return NULL;
}
// 生成密钥对
if (RSA_generate_key_ex(rsa, bits, bne, NULL) != 1) {
printf("Failed to generate RSA key pair.\n");
return NULL;
}
return rsa;
}
```
3. 加载公钥和私钥:
```c
#include <openssl/pem.h>
// 加载公钥
RSA* load_public_key(const char* public_key_file) {
RSA* rsa = RSA_new();
FILE* fp = fopen(public_key_file, "rb");
if (fp == NULL) {
printf("Failed to open public key file.\n");
return NULL;
}
PEM_read_RSA_PUBKEY(fp, &rsa, NULL, NULL);
fclose(fp);
return rsa;
}
// 加载私钥
RSA* load_private_key(const char* private_key_file) {
RSA* rsa = RSA_new();
FILE* fp = fopen(private_key_file, "rb");
if (fp == NULL) {
printf("Failed to open private key file.\n");
return NULL;
}
PEM_read_RSAPrivateKey(fp, &rsa, NULL, NULL);
fclose(fp);
return rsa;
}
```
4. 加密和解密:
```c
#include <openssl/rsa.h>
// RSA 加密
int rsa_encrypt(RSA* rsa, const unsigned char* in, size_t inlen,
unsigned char* out, size_t* outlen) {
int ret = RSA_public_encrypt(inlen, in, out, rsa, RSA_PKCS1_OAEP_PADDING);
if (ret == -1) {
printf("Failed to encrypt.\n");
return 0;
}
*outlen = ret;
return 1;
}
// RSA 解密
int rsa_decrypt(RSA* rsa, const unsigned char* in, size_t inlen,
unsigned char* out, size_t* outlen) {
int ret = RSA_private_decrypt(inlen, in, out, rsa, RSA_PKCS1_OAEP_PADDING);
if (ret == -1) {
printf("Failed to decrypt.\n");
return 0;
}
*outlen = ret;
return 1;
}
```
5. 使用 CRT 加速的 RSA 加密:
```c
// CRT 加速的 RSA 加密
int rsa_crt_encrypt(RSA* rsa, const unsigned char* in, size_t inlen,
unsigned char* out, size_t* outlen) {
int ret = RSA_private_encrypt(inlen, in, out, rsa, RSA_NO_PADDING);
if (ret == -1) {
printf("Failed to CRT-encrypt.\n");
return 0;
}
*outlen = ret;
return 1;
}
```
6. 比较加密和解密的时间:
```c
#include <time.h>
// 比较加密和解密的时间
void compare_rsa_and_crt_rsa(RSA* rsa, const unsigned char* plaintext, size_t plaintext_len) {
clock_t start, end;
double cpu_time_used;
// 普通 RSA 加密和解密
int ret;
unsigned char ciphertext[4096];
size_t ciphertext_len;
unsigned char decryptedtext[4096];
size_t decryptedtext_len;
start = clock();
// 加密
ret = rsa_encrypt(rsa, plaintext, plaintext_len, ciphertext, &ciphertext_len);
if (ret == 0) {
printf("Failed to encrypt.\n");
return;
}
// 解密
ret = rsa_decrypt(rsa, ciphertext, ciphertext_len, decryptedtext, &decryptedtext_len);
if (ret == 0) {
printf("Failed to decrypt.\n");
return;
}
end = clock();
cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
printf("RSA encryption and decryption time: %lf seconds.\n", cpu_time_used);
// CRT 加速的 RSA 加密
unsigned char crt_ciphertext[4096];
size_t crt_ciphertext_len;
start = clock();
// 加密
ret = rsa_crt_encrypt(rsa, plaintext, plaintext_len, crt_ciphertext, &crt_ciphertext_len);
if (ret == 0) {
printf("Failed to CRT-encrypt.\n");
return;
}
end = clock();
cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
printf("CRT-RSA encryption time: %lf seconds.\n", cpu_time_used);
// 比较两种加密方式的结果
if (ciphertext_len != crt_ciphertext_len) {
printf("Encryption results are different.\n");
return;
}
if (memcmp(ciphertext, crt_ciphertext, ciphertext_len) != 0) {
printf("Encryption results are different.\n");
return;
}
}
```
完整的代码实现如下:
阅读全文
相关推荐




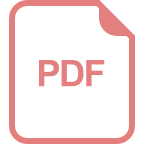
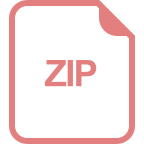
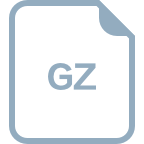
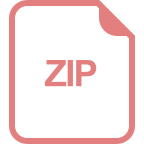
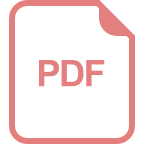
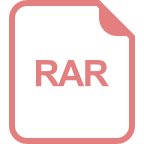
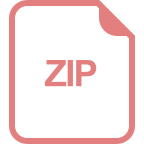
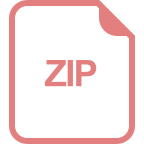
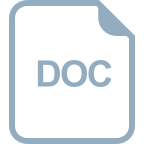
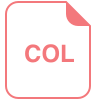

