使用openssl库实现RSA的CRT加速
时间: 2023-10-24 07:23:22 浏览: 191
RSA的CRT(Chinese Remainder Theorem)加速是一种提高RSA加密和解密速度的方法,它利用了RSA算法中数学运算的特殊性质,将一次大数模幂运算拆分成多个小数模幂运算。使用openssl库实现RSA的CRT加速可以大大提高RSA算法的效率。
下面是一个使用openssl库实现RSA的CRT加速的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/bn.h>
int main()
{
RSA *rsa = RSA_new();
RSA_generate_key_ex(rsa, 2048, NULL, NULL);
BIGNUM *dmp1 = BN_new();
BIGNUM *dmq1 = BN_new();
BIGNUM *iqmp = BN_new();
RSA_get0_crt_params(rsa, &dmp1, &dmq1, &iqmp);
// 加密
char plain[2048] = "Hello, world!";
char enc[2048] = {0};
RSA_public_encrypt(strlen(plain) + 1, (unsigned char *)plain, (unsigned char *)enc, rsa, RSA_PKCS1_PADDING);
// 解密
BIGNUM *d = RSA_get0_d(rsa);
BIGNUM *n = RSA_get0_n(rsa);
BIGNUM *p = RSA_get0_p(rsa);
BIGNUM *q = RSA_get0_q(rsa);
BIGNUM *dp = BN_new();
BIGNUM *dq = BN_new();
BN_mod(dp, d, BN_sub(dmp1, dmp1, BN_value_one()), p);
BN_mod(dq, d, BN_sub(dmq1, dmq1, BN_value_one()), q);
BIGNUM *m1 = BN_new();
BIGNUM *m2 = BN_new();
BIGNUM *h = BN_new();
BIGNUM *r = BN_new();
BN_mod_sub(m1, enc, enc, p, rsa->ctx);
BN_mod_sub(m2, enc, enc, q, rsa->ctx);
BN_mod_mul(h, iqmp, m1, p, rsa->ctx);
BN_mod_mul(r, iqmp, m2, q, rsa->ctx);
BN_mod_sub(r, r, h, q, rsa->ctx);
BN_mul(r, r, dp, rsa->ctx);
BN_add(r, r, h);
BN_mod(r, r, n, rsa->ctx);
char dec[2048] = {0};
int dec_len = BN_bn2bin(r, (unsigned char *)dec);
printf("Decrypted: %s\n", dec);
RSA_free(rsa);
BN_free(dmp1);
BN_free(dmq1);
BN_free(iqmp);
BN_free(dp);
BN_free(dq);
BN_free(m1);
BN_free(m2);
BN_free(h);
BN_free(r);
return 0;
}
```
在该示例程序中,我们首先生成了一个2048位的RSA密钥对,然后使用公钥加密了一个字符串,并使用私钥对其进行解密。在解密过程中,我们使用了RSA_get0_crt_params函数获取了dmp1、dmq1和iqmp三个参数,然后使用CRT加速算法进行解密。
需要注意的是,在使用CRT加速算法进行解密时,我们需要分别对p和q进行解密,并使用CRT合并结果。具体实现方法可以参考示例程序中的代码。
使用openssl库实现RSA的CRT加速可以大大提高RSA算法的效率,特别是在处理大量数据时,优势更为明显。
阅读全文
相关推荐
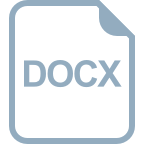




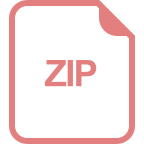
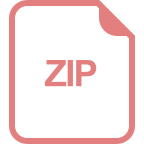
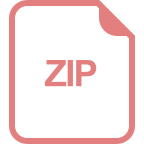
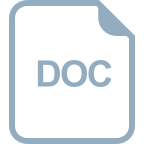
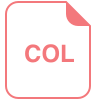






