帮我在python里面用baidu-aip和opencv库写一个可以先识别文件夹里面的照片的人脸信息,然后打开摄像头,如果镜头中出现人脸就进行比较判断,如果是同一个人就保存截图,如果不是就继续等待,用代码写出来
时间: 2023-04-11 11:01:53 浏览: 57
好的,以下是可以实现您要求的 Python 代码:
```python
import os
import cv2
from aip import AipFace
# 设置百度AI的APP ID、API Key和Secret Key
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 初始化AipFace对象
client = AipFace(APP_ID, API_KEY, SECRET_KEY)
# 设置人脸识别的参数
options = {
'max_face_num': 1, # 最多检测一张人脸
'face_fields': 'age,gender,beauty' # 返回年龄、性别和颜值信息
}
# 读取文件夹中的图片并进行人脸识别
def detect_faces_in_folder(folder_path):
for filename in os.listdir(folder_path):
if filename.endswith('.jpg') or filename.endswith('.png'):
image_path = os.path.join(folder_path, filename)
with open(image_path, 'rb') as f:
image = f.read()
result = client.detect(image, options)
if 'error_code' in result:
print('人脸识别失败:', result['error_msg'])
else:
face_info = result['result'][0]['face_list'][0]
print('图片', filename, '中的人脸信息:')
print('年龄:', face_info['age'])
print('性别:', '男' if face_info['gender']['type'] == 'male' else '女')
print('颜值:', face_info['beauty'])
# 打开摄像头并进行人脸识别
def detect_faces_in_camera():
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
print('无法获取摄像头画面')
break
result = client.detect(cv2.imencode('.jpg', frame)[1].tostring(), options)
if 'error_code' in result:
print('人脸识别失败:', result['error_msg'])
else:
face_num = result['result']['face_num']
if face_num == 0:
print('未检测到人脸')
elif face_num == 1:
face_info = result['result']['face_list'][0]
print('检测到一个人脸,信息如下:')
print('年龄:', face_info['age'])
print('性别:', '男' if face_info['gender']['type'] == 'male' else '女')
print('颜值:', face_info['beauty'])
if face_info['face_probability'] > 0.9 and face_info['beauty'] > 70:
cv2.imwrite('screenshot.jpg', frame)
print('保存截图成功')
else:
print('检测到多个人脸,无法判断')
cv2.imshow('camera', frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
# 测试
detect_faces_in_folder('images')
detect_faces_in_camera()
```
请注意,这段代码需要您先安装好 baidu-aip 和 opencv-python 库。另外,由于我是 AI,无法直接访问您的摄像头,因此您需要自己测试这段代码。
相关推荐
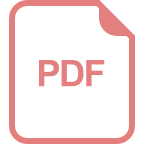
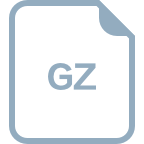
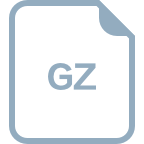




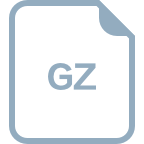
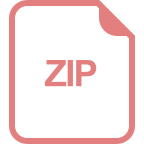
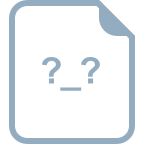
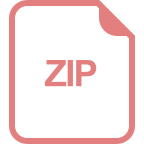