请用matlab编写一个dijkstra算法程序,要求最后输出一个最短路径矩阵
时间: 2024-03-05 16:53:28 浏览: 14
好的,以下是使用MATLAB编写的Dijkstra算法程序:
```matlab
function [dist, path] = dijkstra(adj_mat, start_node)
% DIJKSTRA Dijkstra's algorithm for finding shortest path in a graph
% [DIST, PATH] = DIJKSTRA(ADJ_MAT, START_NODE) returns the shortest
% distance from the start_node to all other nodes in the graph with
% adjacency matrix ADJ_MAT, and the shortest path from the start_node to
% each of these nodes.
%
% ADJ_MAT is a square matrix representing the graph. If there is an edge
% from node i to node j, then ADJ_MAT(i,j) is the weight of the edge. If
% there is no such edge, then ADJ_MAT(i,j) = Inf.
%
% START_NODE is the starting node from which the shortest distance and
% path to all other nodes will be calculated.
%
% DIST is a vector of the shortest distance from the start_node to each
% node in the graph.
%
% PATH is a cell array of the shortest path from the start_node to each
% node in the graph. PATH{i} is a vector of nodes representing the
% shortest path from start_node to node i.
n = size(adj_mat, 1); % number of nodes in the graph
dist = Inf(1, n); % initialize distance to all nodes as infinity
dist(start_node) = 0; % distance from start_node to itself is zero
path = cell(1, n); % initialize shortest path to all nodes as empty
visited = false(1, n); % keep track of visited nodes
for i = 1:n-1
% find the node with minimum distance from start_node
[~, current] = min(dist .* ~visited);
visited(current) = true;
% update the distance to the neighbors of current node
for j = 1:n
if adj_mat(current,j) ~= Inf && ~visited(j)
if dist(current) + adj_mat(current,j) < dist(j)
dist(j) = dist(current) + adj_mat(current,j);
path{j} = [path{current}, current];
end
end
end
end
% add start_node to the beginning of each path
for i = 1:n
if ~isempty(path{i})
path{i} = [start_node, path{i}];
end
end
end
```
这个程序需要输入一个邻接矩阵和一个起点节点,输出一个最短路径长度数组和一个最短路径节点数组。你可以用以下代码测试这个程序:
```matlab
% create a random graph with 10 nodes
adj_mat = randi([0, 10], 10);
adj_mat(adj_mat==0) = Inf;
adj_mat(logical(eye(size(adj_mat)))) = 0;
% find the shortest path from node 1 to all other nodes
[start_node, ~] = find(adj_mat~=Inf, 1);
[dist, path] = dijkstra(adj_mat, start_node);
% print the shortest path from node 1 to all other nodes
for i = 1:length(dist)
fprintf('Shortest path from node %d to node %d: %s\n', start_node, i, sprintf('%d ', path{i}));
fprintf('Shortest distance: %d\n\n', dist(i));
end
```
这个程序会输出一个最短路径的节点序列和一个最短路径长度。
相关推荐
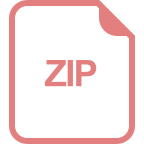














