编写一个程序,当在一个字符串中出现指定子串时就删除它。
时间: 2024-02-12 12:04:41 浏览: 27
好的,我可以为您提供一个 Python 代码示例,用于删除字符串中的指定子串:
```python
def remove_substring(string, substring):
"""删除字符串中的指定子串"""
# 判断字符串是否包含子串
if substring not in string:
return string
# 使用 replace() 方法删除子串
new_string = string.replace(substring, "")
return new_string
```
使用示例:
```python
string = "Hello, World!"
substring = "l"
new_string = remove_substring(string, substring)
print(new_string) # 输出 "Heo, Word!"
```
这个程序会删除字符串中的所有指定子串,如果您只需要删除第一个出现的子串,可以使用 `replace()` 方法的第三个参数来指定替换次数。
相关问题
编写一个程序,当在一个字符串中出现子串时就删除它。
以下是一个使用Python编写的程序,可以实现在一个字符串中删除指定子串的功能:
```python
def delete_substring(string, substring):
while substring in string:
index = string.find(substring)
string = string[:index] + string[index+len(substring):]
return string
```
程序中的 `delete_substring` 函数接收两个参数:`string` 表示原始字符串,`substring` 表示要删除的子串。程序使用一个循环,不断地查找并删除子串,直到原始字符串中不再出现该子串。具体来说,程序使用字符串的 `find` 方法查找子串在原始字符串中的位置,并使用字符串切片操作将子串从原始字符串中删除。
例如,如果我们要删除字符串 `"hello world"` 中的子串 `"o"`,可以这样调用函数:
```python
result = delete_substring("hello world", "o")
print(result) # 输出 "hell wrld"
```
注意,这个程序只能删除字符串中的一个子串。如果要删除多个子串,需要多次调用该函数。
编写一个程序,当在一个字符串中出现子串时就删除它。用C语言
好的,以下是一个简单的 C 语言程序,可以实现删除字符串中指定子串的功能。
```c
#include <stdio.h>
#include <string.h>
void remove_substring(char *str, char *sub) {
int len = strlen(sub);
char *p = str;
while ((p = strstr(p, sub))) {
memmove(p, p + len, strlen(p + len) + 1);
}
}
int main() {
char str[1000], sub[100];
printf("请输入原字符串:");
fgets(str, sizeof(str), stdin);
printf("请输入要删除的子串:");
fgets(sub, sizeof(sub), stdin);
remove_substring(str, sub);
printf("删除后的字符串为:%s", str);
return 0;
}
```
该程序通过使用 `strstr` 函数查找字符串中指定子串的位置,然后使用 `memmove` 函数将子串后面的字符向前移动,最终删除指定子串。
需要注意的是,由于输入的字符串可能包含换行符,因此使用 `fgets` 函数获取字符串输入,并且在输出时需要将换行符去除。
相关推荐
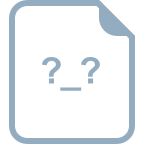
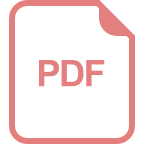
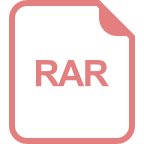
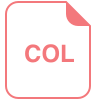
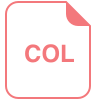
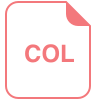
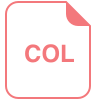








