javafx自定义窗口样式
时间: 2023-10-30 14:05:43 浏览: 75
JavaFX提供了一种简单的方法来自定义窗口样式,可以使用CSS样式表来改变窗口的外观。以下是步骤:
1. 创建一个新的CSS文件,例如“custom.css”。
2. 在CSS文件中定义你想要的样式,例如:
```css
.root {
-fx-background-color: #333;
}
.title-bar {
-fx-background-color: #444;
-fx-text-fill: white;
}
.close-button {
-fx-background-color: #f00;
-fx-text-fill: white;
}
```
3. 在JavaFX应用程序的启动方法中加载CSS文件,并将样式应用到窗口。
```java
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
primaryStage.setTitle("Custom Window Style");
// Load custom CSS style
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("custom.css").toExternalForm());
// Apply custom style to window
primaryStage.initStyle(StageStyle.UNDECORATED);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
4. 在FXML文件中添加一个带有“title-bar”类的Pane,以模拟窗口标题栏。
```xml
<Pane id="title-bar" styleClass="title-bar" onMousePressed="#handleMousePressed" onMouseDragged="#handleMouseDragged">
<Label text="Custom Window Style" />
<Button id="close-button" styleClass="close-button" text="X" onMouseClicked="#handleCloseClicked" />
</Pane>
```
5. 在控制器类中添加处理鼠标事件的方法,使窗口可以拖动和关闭。
```java
public class Controller {
@FXML
private void handleMousePressed(MouseEvent event) {
xOffset = event.getSceneX();
yOffset = event.getSceneY();
}
@FXML
private void handleMouseDragged(MouseEvent event) {
Stage stage = (Stage) ((Node) event.getSource()).getScene().getWindow();
stage.setX(event.getScreenX() - xOffset);
stage.setY(event.getScreenY() - yOffset);
}
@FXML
private void handleCloseClicked(MouseEvent event) {
Stage stage = (Stage) ((Node) event.getSource()).getScene().getWindow();
stage.close();
}
}
```
这样,你就可以使用CSS样式表来创建自定义窗口样式了。注意,需要自己添加拖动和关闭窗口的代码逻辑。
相关推荐
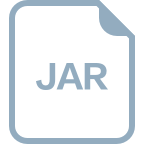
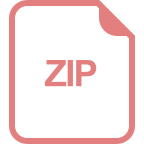














