创建一个文件test.txt,每隔1秒向文件里写入一行数据(使用文件IO编写),使用C语言
时间: 2024-05-24 12:11:39 浏览: 16
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
int main()
{
FILE *fp;
time_t now;
char *time_str;
fp = fopen("test.txt", "w");
if (fp == NULL) {
printf("Error opening file!\n");
exit(1);
}
while (1) {
now = time(NULL);
time_str = ctime(&now);
fprintf(fp, "%s", time_str);
fflush(fp);
sleep(1);
}
fclose(fp);
return 0;
}
相关问题
c语言标准io,将txt文件中某行的某个int类型数据,更改为其他的int类型数据
在C语言中,使用标准IO库可以比较方便地实现将文本文件中某行的某个int类型数据更改为其他的int类型数据。下面是一个示例代码,可以实现将文件中第n行的第m个int类型数据更改为其他的int类型数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
int main()
{
char* filename = "test.txt";
int line_num = 3;
int int_pos = 2; // 第3行的第3个int类型数据
int new_int = 123;
FILE* fp = fopen(filename, "r+");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return 1;
}
char line[MAX_LINE_LEN];
int current_line = 0;
while (fgets(line, MAX_LINE_LEN, fp) != NULL) {
current_line++;
if (current_line == line_num) {
char* token = strtok(line, " ");
int token_count = 0;
while (token != NULL) {
token_count++;
if (token_count == int_pos) {
int int_value = atoi(token);
int value_len = strlen(token);
int int_pos_in_line = (int)(token - line);
fseek(fp, -(strlen(line) - int_pos_in_line), SEEK_CUR);
fprintf(fp, "%d", new_int);
int new_value_len = snprintf(NULL, 0, "%d", new_int);
if (new_value_len > value_len) {
int n_shift = new_value_len - value_len;
char buffer[MAX_LINE_LEN];
while (fgets(buffer, MAX_LINE_LEN, fp) != NULL) {
fseek(fp, -strlen(buffer), SEEK_CUR);
fputs(buffer, fp);
}
ftruncate(fileno(fp), ftell(fp) - n_shift);
}
break;
}
token = strtok(NULL, " ");
}
break;
}
}
if (ferror(fp)) {
printf("Error occurred while reading file %s\n", filename);
fclose(fp);
return 1;
}
fclose(fp);
printf("File %s has been updated.\n", filename);
return 0;
}
```
在以上示例代码中,我们使用了以下标准IO库函数:
- fopen:打开文件。
- fgets:逐行读取文件内容。
- strtok:分隔字符串。
- atoi:将字符串转换为int类型数据。
- fprintf:写入新的int类型数据。
- ftruncate:截断文件大小。
在代码中,我们首先打开文件,并使用fgets函数逐行读取文件内容。当读到需要修改的行时,我们使用strtok函数分隔字符串,并找到需要修改的int类型数据所在的位置。接着,我们使用fprintf函数将新的int类型数据写入到文件中,并使用ftruncate函数截断文件大小(如果新的int类型数据的位数比原来的int类型数据位数多)。最后,我们关闭文件并输出更新成功的信息。
需要注意的是,以上示例代码中并没有处理文件中行末的换行符,如果需要更精细的处理可以在代码中加以修改。同时,如果文件中存在非法的int类型数据,以上代码可能会出现错误。
c语言标准io更改文件某行内容
在C语言中,标准IO库提供了一系列函数用于文件的读写操作,其中包括了更改文件某行的内容的函数。
具体操作步骤如下:
1. 打开文件:使用fopen()函数打开需要修改的文件,以"w+"模式打开,同时检查是否打开成功。
2. 定位到需要修改的行:使用fseek()函数将文件指针定位到需要修改的行的起始位置,可以通过读取每行的字符数和行数来实现。
3. 更改行内容:使用fprintf()函数将需要修改的内容写入到文件中,覆盖原有内容。
4. 关闭文件:使用fclose()函数关闭文件。
下面是一个示例代码,可以更改文件的第n行内容:
```c
#include <stdio.h>
int main() {
FILE *fp;
int n = 3; // 需要修改的行数
char line[100];
fp = fopen("test.txt", "w+");
if (fp == NULL) {
printf("打开文件失败\n");
return 1;
}
// 将文件指针定位到需要修改的行
for (int i = 1; i < n; i++) {
fgets(line, sizeof(line), fp);
}
// 将需要修改的内容写入文件
fprintf(fp, "This is the new content.\n");
fclose(fp);
return 0;
}
```
注意事项:
1. 如果需要修改文件中的多个行,可以使用循环来实现。
2. 在修改文件内容时,需要确保修改后的内容与原有内容的长度相等,否则可能会出现文件大小不一致的问题。
相关推荐
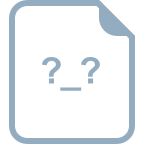
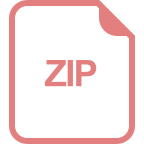








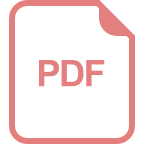
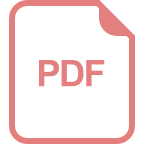
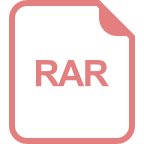