写一段python代码将yolo格式转为voc格式
时间: 2023-09-05 15:04:43 浏览: 271
### 回答1:
import os
import shutil
import glob#源数据路径
yolo_path = './yolo_data'
#目标路径
voc_path = './voc_data'
#检查路径是否存在,不存在则新建
if not os.path.exists(voc_path):
os.mkdir(voc_path)
#获取所有源数据文件
yolo_list = glob.glob(os.path.join(yolo_path, '*'))
#检查每一个源文件
for yolo_file in yolo_list:
#获取文件名
basename = os.path.basename(yolo_file)
#获取文件名和后缀
basename, ext = os.path.splitext(basename)
#拼接输出文件路径
voc_file = os.path.join(voc_path, basename + '.xml')
#打开文件
with open(yolo_file) as f:
#按行读取
lines = f.readlines()
#检查文件是否为空
if len(lines) == 0:
continue
#读取每一行内容
for line in lines:
#按空格分割
line_list = line.split(' ')
#获取标签
label = line_list[0]
#获取坐标
xmin = line_list[1]
ymin = line_list[2]
xmax = line_list[3]
ymax = line_list[4]
#创建voc格式文件
with open(voc_file, 'w') as fw:
fw.write('<annotation>\n')
fw.write('\t<filename>{}.jpg</filename>\n'.format(basename))
fw.write('\t<size>\n')
fw.write('\t\t<width>1920</width>\n')
fw.write('\t\t<height>1080</height>\n')
fw.write('\t\t<depth>3</depth>\n')
fw.write('\t</size>\n')
fw.write('\t<object>\n')
fw.write('\t\t<name>{}</name>\n'.format(label))
fw.write('\t\t<bndbox>\n')
fw.write('\t\t\t<xmin>{}</xmin>\n'.format(xmin))
fw.write('\t\t\t<ymin>{}</ymin>\n'.format(ymin))
fw.write('\t\t\t<xmax>{}</xmax>\n'.format(xmax))
fw.write('\t\t\t<ymax>{}</ymax>\n'.format(ymax))
fw.write('\t\t</bndbox>\n')
fw.write('\t</object>\n')
fw.write('</annotation>\n')
#复制图片
shutil.copy(os.path.join(yolo_path, basename+'.jpg'), voc_path)
### 回答2:
YOLO格式和VOC格式是两种用于物体检测的标注格式。下面是一个用Python编写的将YOLO格式转换为VOC格式的简单代码示例:
```python
import xml.etree.ElementTree as ET
def yolo_to_voc(yolo_path, output_path):
with open(yolo_path, 'r') as yolo_file:
lines = yolo_file.readlines()
root = ET.Element("annotation")
folder = ET.SubElement(root, "folder")
folder.text = "VOC2007"
filename = ET.SubElement(root, "filename")
filename.text = "image.jpg"
size = ET.SubElement(root, "size")
width = ET.SubElement(size, "width")
width.text = "416" # 替换为图片的宽度
height = ET.SubElement(size, "height")
height.text = "416" # 替换为图片的高度
depth = ET.SubElement(size, "depth")
depth.text = "3"
for line in lines:
label, x_center, y_center, w, h = line.split()
object = ET.SubElement(root, "object")
name = ET.SubElement(object, "name")
name.text = label
pose = ET.SubElement(object, "pose")
pose.text = "Unspecified"
truncated = ET.SubElement(object, "truncated")
truncated.text = "0"
difficult = ET.SubElement(object, "difficult")
difficult.text = "0"
bndbox = ET.SubElement(object, "bndbox")
xmin = ET.SubElement(bndbox, "xmin")
xmin.text = str(int(float(x_center) - (float(w) / 2.0)))
ymin = ET.SubElement(bndbox, "ymin")
ymin.text = str(int(float(y_center) - (float(h) / 2.0)))
xmax = ET.SubElement(bndbox, "xmax")
xmax.text = str(int(float(x_center) + (float(w) / 2.0)))
ymax = ET.SubElement(bndbox, "ymax")
ymax.text = str(int(float(y_center) + (float(h) / 2.0)))
tree = ET.ElementTree(root)
tree.write(output_path)
yolo_path = "path/to/yolo.txt" # 替换为YOLO格式标注文件的路径
output_path = "path/to/output.xml" # 替换为转换后的VOC格式标注文件的保存路径
yolo_to_voc(yolo_path, output_path)
```
在运行代码之前,请替换示例中的参量`yolo_path`和`output_path`为正确的文件路径。这段代码会将YOLO格式的标注文件转换成VOC格式,并将输出保存为一个XML文件。请确保YOLO格式的标注文件包含类别标签、边界框的中心坐标、宽度和高度信息。
### 回答3:
下面是一个将YOLO格式转换为VOC格式的Python代码段:
```python
import os
import xml.etree.ElementTree as ET
def convert_yolo_to_voc(yolo_file, voc_dir):
# 读取YOLO格式文件
with open(yolo_file, 'r') as f:
lines = f.readlines()
for line in lines:
line = line.strip().split()
image_name = line[0]
labels = line[1:]
# 创建VOC格式的XML文件
root = ET.Element("annotation")
folder = ET.SubElement(root, "folder")
folder.text = voc_dir
filename = ET.SubElement(root, "filename")
filename.text = image_name
for label in labels:
obj = ET.SubElement(root, "object")
name = ET.SubElement(obj, "name")
name.text = label
# 保存VOC格式的XML文件
xml_path = os.path.join(voc_dir, os.path.splitext(image_name)[0] + ".xml")
tree = ET.ElementTree(root)
tree.write(xml_path)
print("转换完成!")
# 调用示例
yolo_file = "path/to/yolo.txt"
voc_dir = "path/to/voc"
convert_yolo_to_voc(yolo_file, voc_dir)
```
请将代码中的路径替换为实际路径。该代码会读取YOLO格式的文件(假设为"yolo.txt"),将每行的第一个值作为图像名称,后续值作为标签,然后将其转换为对应的VOC格式的XML文件,存储在指定的目录(假设为"path/to/voc")中。
阅读全文
相关推荐
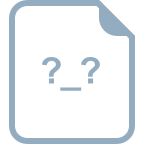
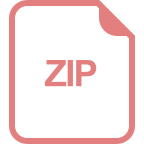
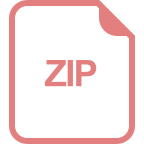
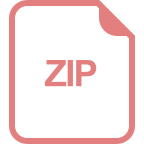
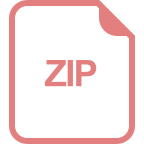
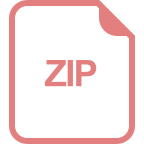
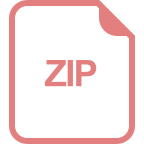
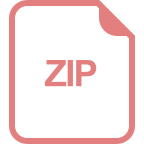
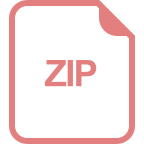
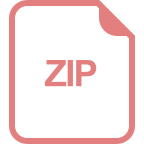
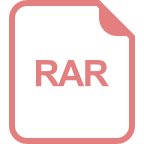
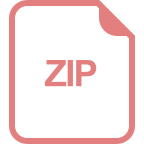
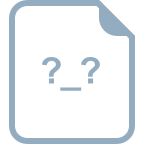
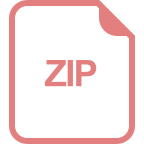
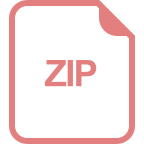