c语言用栈实现计算器(中缀表达式)
时间: 2023-08-02 08:14:23 浏览: 106
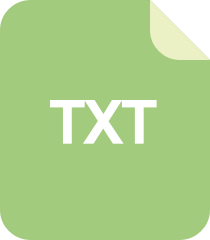
中缀表达式转化为后缀表达式
栈是实现计算器的核心数据结构,可以用来存储运算符和操作数。下面是使用栈实现中缀表达式计算器的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <ctype.h>
#define MAX_SIZE 50
typedef struct {
int top;
int data[MAX_SIZE];
} Stack;
void push(Stack *s, int value) {
if (s->top == MAX_SIZE - 1) {
printf("Stack Overflow\n");
return;
}
s->data[++(s->top)] = value;
}
int pop(Stack *s) {
if (s->top == -1) {
printf("Stack Underflow\n");
exit(1);
}
return s->data[(s->top)--];
}
bool is_operator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
int get_priority(char op) {
switch (op) {
case '(':
return 0;
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return -1;
}
}
int calculate(int op1, int op2, char operator) {
switch (operator) {
case '+':
return op1 + op2;
case '-':
return op1 - op2;
case '*':
return op1 * op2;
case '/':
return op1 / op2;
default:
return -1;
}
}
int evaluate_expression(char *expression) {
Stack operand_stack;
Stack operator_stack;
operand_stack.top = -1;
operator_stack.top = -1;
int i = 0;
while (expression[i] != '\0') {
if (isdigit(expression[i])) {
int operand = 0;
while (isdigit(expression[i])) {
operand = operand * 10 + (expression[i] - '0');
i++;
}
push(&operand_stack, operand);
} else if (is_operator(expression[i])) {
while (operator_stack.top >= 0 && get_priority(expression[i]) <= get_priority(operator_stack.data[operator_stack.top])) {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
int result = calculate(op1, op2, op);
push(&operand_stack, result);
}
push(&operator_stack, expression[i]);
i++;
} else if (expression[i] == '(') {
push(&operator_stack, expression[i]);
i++;
} else if (expression[i] == ')') {
while (operator_stack.data[operator_stack.top] != '(') {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
int result = calculate(op1, op2, op);
push(&operand_stack, result);
}
pop(&operator_stack);
i++;
} else {
i++;
}
}
while (operator_stack.top >= 0) {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
int result = calculate(op1, op2, op);
push(&operand_stack, result);
}
return pop(&operand_stack);
}
int main() {
char str[MAX_SIZE];
printf("Enter expression: ");
scanf("%s", str);
int result = evaluate_expression(str);
printf("Result: %d\n", result);
return 0;
}
```
这个程序支持加、减、乘、除和括号,可以计算包括负数在内的表达式。你可以在控制台中输入一个表达式,程序会输出计算结果。
阅读全文
相关推荐
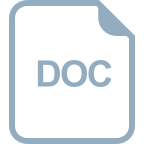
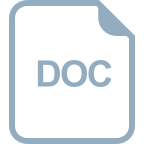
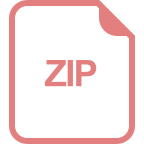

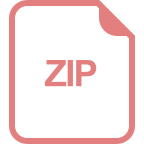
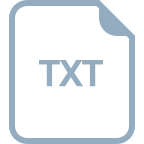
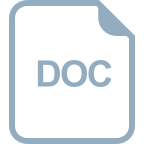
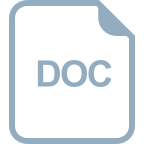

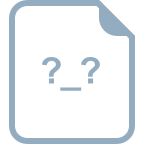
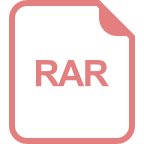
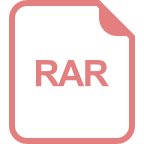
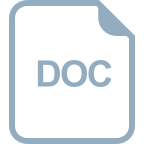
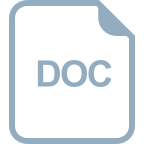
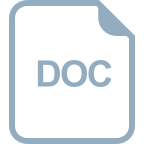

