Hadoop编写矩阵乘法的实例
时间: 2024-05-13 11:16:02 浏览: 206
Hadoop编写矩阵乘法的实例主要分为三个步骤:
1. Map阶段:将矩阵A和矩阵B分别划分为若干个小块,每个Map任务负责计算其中的一块矩阵乘积,并将结果输出为键值对。
2. Shuffle阶段:将所有Map任务输出的键值对按照键进行排序并分组,确保相同的键(即矩阵乘积的行和列)被分到同一个Reduce任务中。
3. Reduce阶段:将每个Reduce任务分别计算相同键的值的和,即计算矩阵乘积中某个位置的值,并将结果输出。
下面是Hadoop编写矩阵乘法的示例代码:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class MatrixMultiplication {
public static class MatrixMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
private int matrixSize = 2; // 矩阵大小为2x2
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String[] line = value.toString().split(",");
if (line[0].equals("A")) {
for (int i = 0; i < matrixSize; i++) {
outKey.set(line[1] + "," + i);
outValue.set(line[0] + "," + line[2] + "," + line[3]);
context.write(outKey, outValue);
}
} else {
for (int i = 0; i < matrixSize; i++) {
outKey.set(i + "," + line[2]);
outValue.set(line[0] + "," + line[1] + "," + line[3]);
context.write(outKey, outValue);
}
}
}
}
public static class MatrixReducer extends Reducer<Text, Text, Text, IntWritable> {
public void reduce(Text key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
int[] a = new int[2];
int[] b = new int[2];
for (Text val : values) {
String[] line = val.toString().split(",");
if (line[0].equals("A")) {
a[Integer.parseInt(line[1])] = Integer.parseInt(line[2]);
} else {
b[Integer.parseInt(line[1])] = Integer.parseInt(line[2]);
}
}
int result = a[0] * b[0] + a[1] * b[1];
context.write(key, new IntWritable(result));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "matrix multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在上述代码中,MatrixMapper类实现了Map阶段的逻辑,将输入的矩阵A和矩阵B分别转换为以列或行为键的键值对,并将矩阵乘积的值作为值进行输出。
MatrixReducer类实现了Reduce阶段的逻辑,将相同键的值进行累加,并计算矩阵乘积的结果。
在main函数中,设置了Mapper和Reducer类,并指定输入和输出路径,最后启动了MapReduce任务。
阅读全文
相关推荐
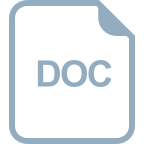
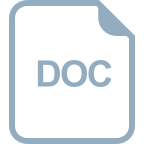
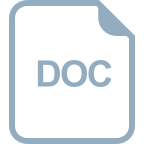



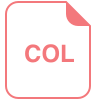
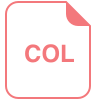
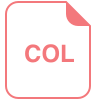
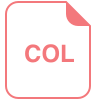
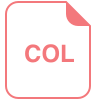
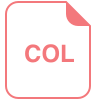
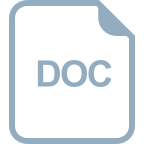
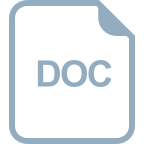
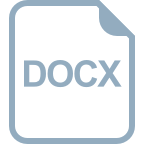
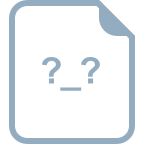