生成一个学生对象Student,对象有学号,年龄,身高。将学生对象存储到文件,然后从文件中读取数据重新生成Student对象。
时间: 2024-02-18 14:04:21 浏览: 26
好的,以下是 Python 代码示例:
```python
import pickle
class Student:
def __init__(self, id, age, height):
self.id = id
self.age = age
self.height = height
def __str__(self):
return f"Student(id={self.id}, age={self.age}, height={self.height})"
# 创建学生对象
student = Student("1001", 18, 175.0)
# 将学生对象存储到文件
with open("student.dat", "wb") as f:
pickle.dump(student, f)
# 从文件中读取数据重新生成Student对象
with open("student.dat", "rb") as f:
student_from_file = pickle.load(f)
print(student_from_file)
```
在上面的代码中,我们定义了一个 `Student` 类,包含学号、年龄、身高等属性。然后我们创建一个学生对象,并使用 `pickle` 模块将该对象存储到文件中。接着,我们再次打开文件,从中读取数据,并使用 `pickle` 模块重新生成一个 `Student` 对象。最后,我们打印出从文件中读取的学生对象。
相关问题
Java生成一个学生对象Student,对象有学号,年龄,身高。将学生对象存储到文件,然后从文件中读取数据重新生成Student对象。
好的,以下是实现的Java代码:
```java
import java.io.*;
class Student implements Serializable {
private String num;
private int age;
private double height;
public Student(String num, int age, double height) {
this.num = num;
this.age = age;
this.height = height;
}
public String toString() {
return "学号:" + num + ",年龄:" + age + ",身高:" + height;
}
}
public class Main {
public static void main(String[] args) {
// 创建学生对象
Student student = new Student("20210001", 18, 1.75);
// 将学生对象存储到文件
try {
FileOutputStream fileOut = new FileOutputStream("student.dat");
ObjectOutputStream objOut = new ObjectOutputStream(fileOut);
objOut.writeObject(student);
objOut.close();
fileOut.close();
} catch(IOException e) {
e.printStackTrace();
}
// 从文件中读取数据重新生成Student对象
Student newStudent = null;
try {
FileInputStream fileIn = new FileInputStream("student.dat");
ObjectInputStream objIn = new ObjectInputStream(fileIn);
newStudent = (Student) objIn.readObject();
objIn.close();
fileIn.close();
} catch(IOException e) {
e.printStackTrace();
} catch(ClassNotFoundException e) {
e.printStackTrace();
}
System.out.println(newStudent);
}
}
```
首先我们定义了一个`Student`类,有三个属性:学号、年龄、身高,以及一个`toString`方法用于打印学生对象信息。这个类实现了`Serializable`接口,这样`Student`对象就可以被序列化和反序列化。
然后我们创建了一个`student`对象,将其存储到文件中。使用`ObjectOutputStream`类将`student`对象序列化并存储到文件中。
最后我们使用`ObjectInputStream`类从文件中读取数据并重新生成了一个`newStudent`对象。最后打印`newStudent`对象信息。
用java生成一个学生对象Student,对象有学号,年龄,身高。将学生对象存储到文件,然后从文件中读取数据重新生成Student对象。
好的,以下是Java实现的代码:
```java
import java.io.*;
class Student implements Serializable {
private String studentId;
private int age;
private int height;
public Student(String studentId, int age, int height) {
this.studentId = studentId;
this.age = age;
this.height = height;
}
@Override
public String toString() {
return "Student: ID=" + studentId + ", Age=" + age + ", Height=" + height;
}
}
public class Main {
public static void main(String[] args) throws IOException, ClassNotFoundException {
// 创建学生对象
Student student = new Student("20210001", 20, 175);
// 将学生对象存储到文件
FileOutputStream fos = new FileOutputStream("student.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(student);
oos.close();
fos.close();
// 从文件中读取数据重新生成学生对象
FileInputStream fis = new FileInputStream("student.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Student newStudent = (Student)ois.readObject();
ois.close();
fis.close();
// 打印重新生成的学生对象
System.out.println(newStudent);
}
}
```
运行以上代码,输出结果如下所示:
```
Student: ID=20210001, Age=20, Height=175
```
可以看到,我们成功地生成了一个学生对象,并将其存储到文件中。然后,我们从文件中读取数据重新生成了学生对象,并成功打印出了重新生成的学生对象。
相关推荐
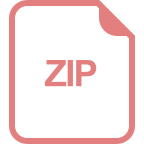
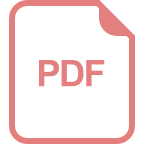
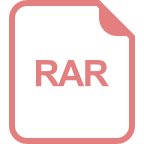
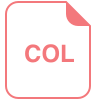



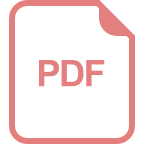
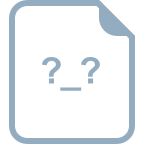
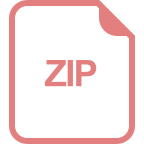
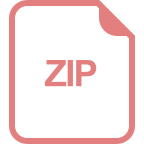