SHA256 C语言代码
时间: 2023-11-12 13:48:36 浏览: 176
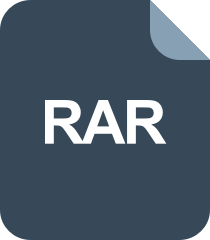
sha256sum c语言代码

以下是SHA256 C语言代码的基本实现:
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
#define ROTR(x,n) (((x) >> (n)) | ((x) << (32 - (n))))
#define ROTL(x,n) (((x) << (n)) | ((x) >> (32 - (n))))
#define SHR(x,n) ((x) >> (n))
#define CH(x,y,z) (((x) & (y)) ^ (~(x) & (z)))
#define MAJ(x,y,z) (((x) & (y)) ^ ((x) & (z)) ^ ((y) & (z)))
#define EP0(x) (ROTR(x,2) ^ ROTR(x,13) ^ ROTR(x,22))
#define EP1(x) (ROTR(x,6) ^ ROTR(x,11) ^ ROTR(x,25))
#define SIG0(x) (ROTR(x,7) ^ ROTR(x,18) ^ SHR(x,3))
#define SIG1(x) (ROTR(x,17) ^ ROTR(x,19) ^ SHR(x,10))
#define SHA256_BLOCK_SIZE 64
const uint32_t K[] = {
0x428a2f98, 0x71374491, 0xb5c0fbcf, 0xe9b5dba5,
0x3956c25b, 0x59f111f1, 0x923f82a4, 0xab1c5ed5,
0xd807aa98, 0x12835b01, 0x243185be, 0x550c7dc3,
0x72be5d74, 0x80deb1fe, 0x9bdc06a7, 0xc19bf174,
0xe49b69c1, 0xefbe4786, 0x0fc19dc6, 0x240ca1cc,
0x2de92c6f, 0x4a7484aa, 0x5cb0a9dc, 0x76f988da,
0x983e5152, 0xa831c66d, 0xb00327c8, 0xbf597fc7,
0xc6e00bf3, 0xd5a79147, 0x06ca6351, 0x14292967,
0x27b70a85, 0x2e1b2138, 0x4d2c6dfc, 0x53380d13,
0x650a7354, 0x766a0abb, 0x81c2c92e, 0x92722c85,
0xa2bfe8a1, 0xa81a664b, 0xc24b8b70, 0xc76c51a3,
0xd192e819, 0xd6990624, 0xf40e3585, 0x106aa070,
0x19a4c116, 0x1e376c08, 0x2748774c, 0x34b0bcb5,
0x391c0cb3, 0x4ed8aa4a, 0x5b9cca4f, 0x682e6ff3,
0x748f82ee, 0x78a5636f, 0x84c87814, 0x8cc70208,
0x90befffa, 0xa4506ceb, 0xbef9a3f7, 0xc67178f2
};
void sha256_transform(uint32_t state[], const uint8_t data[]) {
uint32_t W[64];
uint32_t A, B, C, D, E, F, G, H, T1, T2;
int i;
for (i = 0; i < 16; i++) {
W[i] = ((uint32_t)data[i * 4] << 24) |
((uint32_t)data[i * 4 + 1] << 16) |
((uint32_t)data[i * 4 + 2] << 8) |
((uint32_t)data[i * 4 + 3]);
}
for (i = 16; i < 64; i++) {
W[i] = SIG1(W[i - 2]) + W[i - 7] + SIG0(W[i - 15]) + W[i - 16];
}
A = state[0];
B = state[1];
C = state[2];
D = state[3];
E = state[4];
F = state[5];
G = state[6];
H = state[7];
for (i = 0; i < 64; i++) {
T1 = H + EP1(E) + CH(E,F,G) + K[i] + W[i];
T2 = EP0(A) + MAJ(A,B,C);
H = G;
G = F;
F = E;
E = D + T1;
D = C;
C = B;
B = A;
A = T1 + T2;
}
state[0] += A;
state[1] += B;
state[2] += C;
state[3] += D;
state[4] += E;
state[5] += F;
state[6] += G;
state[7] += H;
}
void sha256_init(uint32_t state[]) {
state[0] = 0x6a09e667;
state[1] = 0xbb67ae85;
state[2] = 0x3c6ef372;
state[3] = 0xa54ff53a;
state[4] = 0x510e527f;
state[5] = 0x9b05688c;
state[6] = 0x1f83d9ab;
state[7] = 0x5be0cd19;
}
void sha256_update(uint32_t state[], uint32_t *bitlen, const uint8_t data[], size_t len) {
uint32_t i, j;
for (i = 0; i < len; i++) {
state[8] += data[i];
state[8] &= 0xff;
if (++(*bitlen) == 512) {
sha256_transform(state, &data[i - 63]);
*bitlen = 0;
}
}
j = i;
if (*bitlen > 447) {
for (; i < 512 / 8; i++) {
state[8] += 0;
state[8] &= 0xff;
}
sha256_transform(state, &data[j - 63]);
memset(state, 0, 8 * sizeof(uint32_t));
}
for (; i < (512 - 64) / 8; i++) {
state[8] += 0;
state[8] &= 0xff;
}
uint64_t bitlen_tmp = (*bitlen) << 3;
uint8_t *p = (uint8_t *)&bitlen_tmp;
for (; i < 512 / 8; i++) {
state[8] += p[7 - i % 8];
state[8] &= 0xff;
}
sha256_transform(state, &data[j - 63]);
}
void sha256_final(uint32_t state[], uint32_t *bitlen, uint8_t hash[]) {
int i;
sha256_update(state, bitlen, (uint8_t *)"\x80", 1);
while (*bitlen != 448) {
sha256_update(state, bitlen, (uint8_t *)"\x00", 1);
}
uint64_t bitlen_tmp = (*bitlen) << 3;
uint8_t *p = (uint8_t *)&bitlen_tmp;
for (i = 0; i < 8; i++) {
sha256_update(state, bitlen, &p[7 - i], 1);
}
for (i = 0; i < 8; i++) {
hash[4 * i] = (state[i] >> 24) & 0xff;
hash[4 * i + 1] = (state[i] >> 16) & 0xff;
hash[4 * i + 2] = (state[i] >> 8) & 0xff;
hash[4 * i + 3] = state[i] & 0xff;
}
}
void sha256(const uint8_t data[], size_t len, uint8_t hash[]) {
uint32_t state[8];
uint32_t bitlen = 0;
sha256_init(state);
sha256_update(state, &bitlen, data, len);
sha256_final(state, &bitlen, hash);
}
int main() {
uint8_t data[] = "hello world";
uint8_t hash[32];
sha256(data, strlen((char *)data), hash);
for (int i = 0; i < 32; i++) {
printf("%02x", hash[i]);
}
printf("\n");
return 0;
}
```
这个代码可以对输入的数据计算出其SHA256哈希值,并输出到控制台上。但需要注意,该实现并不是最优的,仅供参考。在实际应用中,应该使用更加优化的实现。
阅读全文
相关推荐
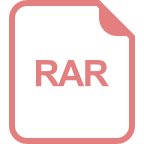
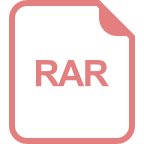

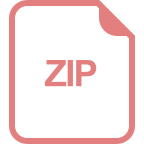




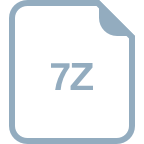





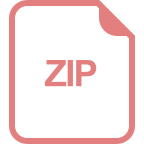