生成SHA256的C语言代码
时间: 2023-03-31 20:05:05 浏览: 65
可以使用openssl库中的SHA256函数来生成SHA256的C语言代码。具体实现可以参考以下代码:
#include <stdio.h>
#include <openssl/sha.h>
int main() {
unsigned char input[] = "hello world";
unsigned char output[SHA256_DIGEST_LENGTH];
SHA256(input, sizeof(input) - 1, output);
for(int i = ; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", output[i]);
}
return ;
}
相关问题
c语言编写sha256代码
以下是使用C语言编写SHA256代码的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#define ROTRIGHT(word,bits) (((word) >> (bits)) | ((word) << (32 - (bits))))
#define SHA256_BLOCK_SIZE 32 // SHA256 outputs a 32 byte digest
// struct to hold SHA256 context
typedef struct {
uint32_t total[2];
uint32_t state[8];
uint8_t buffer[64];
} SHA256_CTX;
// array of SHA256 constants
static const uint32_t K[] = {
0x428a2f98, 0x71374491, 0xb5c0fbcf, 0xe9b5dba5,
0x3956c25b, 0x59f111f1, 0x923f82a4, 0xab1c5ed5,
0xd807aa98, 0x12835b01, 0x243185be, 0x550c7dc3,
0x72be5d74, 0x80deb1fe, 0x9bdc06a7, 0xc19bf174,
0xe49b69c1, 0xefbe4786, 0x0fc19dc6, 0x240ca1cc,
0x2de92c6f, 0x4a7484aa, 0x5cb0a9dc, 0x76f988da,
0x983e5152, 0xa831c66d, 0xb00327c8, 0xbf597fc7,
0xc6e00bf3, 0xd5a79147, 0x06ca6351, 0x14292967,
0x27b70a85, 0x2e1b2138, 0x4d2c6dfc, 0x53380d13,
0x650a7354, 0x766a0abb, 0x81c2c92e, 0x92722c85,
0xa2bfe8a1, 0xa81a664b, 0xc24b8b70, 0xc76c51a3,
0xd192e819, 0xd6990624, 0xf40e3585, 0x106aa070,
0x19a4c116, 0x1e376c08, 0x2748774c, 0x34b0bcb5,
0x391c0cb3, 0x4ed8aa4a, 0x5b9cca4f, 0x682e6ff3,
0x748f82ee, 0x78a5636f, 0x84c87814, 0x8cc70208,
0x90befffa, 0xa4506ceb, 0xbef9a3f7, 0xc67178f2
};
// function to initialize SHA256 context
void sha256_init(SHA256_CTX *ctx) {
ctx->total[0] = 0;
ctx->total[1] = 0;
ctx->state[0] = 0x6a09e667;
ctx->state[1] = 0xbb67ae85;
ctx->state[2] = 0x3c6ef372;
ctx->state[3] = 0xa54ff53a;
ctx->state[4] = 0x510e527f;
ctx->state[5] = 0x9b05688c;
ctx->state[6] = 0x1f83d9ab;
ctx->state[7] = 0x5be0cd19;
}
// function to perform SHA256 transformation
void sha256_transform(SHA256_CTX *ctx, const uint8_t *data) {
uint32_t a, b, c, d, e, f, g, h, i, j, t1, t2, m[64];
// convert data into 32-bit words
for (i = 0, j = 0; i < 16; ++i, j += 4) {
m[i] = ((uint32_t)data[j] << 24) | ((uint32_t)data[j + 1] << 16) |
((uint32_t)data[j + 2] << 8) | ((uint32_t)data[j + 3]);
}
// expand the 16 32-bit words into 64 32-bit words
for ( ; i < 64; ++i) {
m[i] = m[i - 16] + ROTRIGHT(m[i - 15], 7) + m[i - 7] + ROTRIGHT(m[i - 2], 17);
}
// initialize hash values
a = ctx->state[0];
b = ctx->state[1];
c = ctx->state[2];
d = ctx->state[3];
e = ctx->state[4];
f = ctx->state[5];
g = ctx->state[6];
h = ctx->state[7];
// compression function main loop
for (i = 0; i < 64; ++i) {
t1 = h + (ROTRIGHT(e, 6) ^ ROTRIGHT(e, 11) ^ ROTRIGHT(e, 25)) + ((e & f) ^ (~e & g)) + K[i] + m[i];
t2 = (ROTRIGHT(a, 2) ^ ROTRIGHT(a, 13) ^ ROTRIGHT(a, 22)) + ((a & b) ^ (a & c) ^ (b & c));
h = g;
g = f;
f = e;
e = d + t1;
d = c;
c = b;
b = a;
a = t1 + t2;
}
// add the compressed chunk to the current hash value
ctx->state[0] += a;
ctx->state[1] += b;
ctx->state[2] += c;
ctx->state[3] += d;
ctx->state[4] += e;
ctx->state[5] += f;
ctx->state[6] += g;
ctx->state[7] += h;
}
// function to update SHA256 context with new data
void sha256_update(SHA256_CTX *ctx, const uint8_t *data, uint32_t length) {
uint32_t i = length & 0x3f;
uint32_t j = 0;
ctx->total[0] += length;
if (ctx->total[0] < length) {
ctx->total[1]++;
}
if (i + length >= 64) {
memcpy(&ctx->buffer[i], data, 64 - i);
sha256_transform(ctx, ctx->buffer);
for (j = 0; j + 63 < length - i; j += 64) {
sha256_transform(ctx, &data[i + j]);
}
i = 0;
}
memcpy(&ctx->buffer[i], &data[j], length - j);
}
// function to finalize SHA256 context and generate digest
void sha256_final(SHA256_CTX *ctx, uint8_t *digest) {
uint8_t i = 0;
uint32_t j = 0;
// pad the last chunk
i = ctx->total[0] & 0x3f;
ctx->buffer[i++] = 0x80;
if (i + 8 > 64) {
memset(&ctx->buffer[i], 0, 64 - i);
sha256_transform(ctx, ctx->buffer);
i = 0;
}
memset(&ctx->buffer[i], 0, 64 - i);
ctx->total[0] *= 8;
ctx->buffer[56] = (ctx->total[0] >> 24) & 0xff;
ctx->buffer[57] = (ctx->total[0] >> 16) & 0xff;
ctx->buffer[58] = (ctx->total[0] >> 8) & 0xff;
ctx->buffer[59] = ctx->total[0] & 0xff;
ctx->total[1] *= 8;
ctx->buffer[60] = (ctx->total[1] >> 24) & 0xff;
ctx->buffer[61] = (ctx->total[1] >> 16) & 0xff;
ctx->buffer[62] = (ctx->total[1] >> 8) & 0xff;
ctx->buffer[63] = ctx->total[1] & 0xff;
sha256_transform(ctx, ctx->buffer);
// output hash value as 32 byte binary string
for (i = 0, j = 0; i < 8; ++i, j += 4) {
digest[j] = (ctx->state[i] >> 24) & 0xff;
digest[j + 1] = (ctx->state[i] >> 16) & 0xff;
digest[j + 2] = (ctx->state[i] >> 8) & 0xff;
digest[j + 3] = ctx->state[i] & 0xff;
}
}
// function to compute SHA256 digest of input data
void sha256_compute(const uint8_t *data, uint32_t length, uint8_t *digest) {
SHA256_CTX ctx;
sha256_init(&ctx);
sha256_update(&ctx, data, length);
sha256_final(&ctx, digest);
}
// sample usage
int main() {
uint8_t data[] = "hello world";
uint8_t digest[SHA256_BLOCK_SIZE];
sha256_compute(data, strlen(data), digest);
printf("SHA256 digest: ");
for (int i = 0; i < SHA256_BLOCK_SIZE; ++i) {
printf("%02x", digest[i]);
}
printf("\n");
return 0;
}
```
在上面的代码中,使用了SHA256_CTX结构体来存储SHA256上下文,包括总字节数、状态和缓冲区。sha256_init用于初始化上下文,sha256_update用于将新数据更新到上下文中,sha256_transform用于执行SHA256转换,sha256_final用于最终化上下文并生成摘要,sha256_compute用于计算输入数据的SHA256摘要。最后,我们在主函数中对“hello world”字符串进行SHA256哈希,并将结果打印出来。
c语言 sha256
C语言中的SHA-256是一种安全哈希算法,用于生成消息摘要。它将任意长度的消息作为输入,产生一个256位的哈希值作为输出。SHA-256算法是一种密码学安全哈希函数,被广泛应用于数字签名、消息认证、随机数生成等领域。在C语言中,可以通过引用sha256.h头文件并调用其中的sha256函数来实现SHA-256算法的计算。在引用的代码中,main函数中的代码演示了如何使用sha256函数计算一个字符串的SHA-256哈希值,并将结果输出到控制台上。
相关推荐
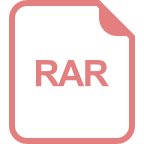
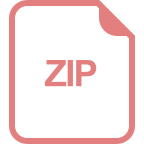
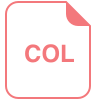











