@GetMapping("/selectCode/{role}") public JsonData<User> selectCode(@PathVariable Integer role, @RequestBody Page page) 请问前端vue如何调用该接口?
时间: 2024-03-17 07:40:34 浏览: 44
前端Vue可以使用axios库发送HTTP请求来调用该接口。首先需要在Vue组件中引入axios库,可以在`<script>`标签中添加以下代码:
```
import axios from 'axios';
```
然后在需要调用该接口的方法中,可以使用axios库发送GET请求来调用接口。例如:
```
getData() {
axios.get('/selectCode/' + this.role, {
params: {
page: this.page
}
}).then(response => {
// 处理响应数据
}).catch(error => {
// 处理错误
});
}
```
在上面的代码中,`this.role` 表示从Vue组件中获取的 `role` 参数,`this.page` 表示从Vue组件中获取的 `page` 参数。`axios.get()` 方法用于发送GET请求,第一个参数是接口的URL,第二个参数是一个配置对象,其中 `params` 属性表示要传递给接口的参数。当接口调用成功时,`then()` 方法将会被执行,可以在里面处理响应数据;当接口调用失败时,`catch()` 方法将会被执行,可以在里面处理错误。
相关问题
@GetMapping("/comments/{page}/{size}") public ApiResult<IPage<CommentVO>> findAll(@PathVariable("page") Integer page, @PathVariable("size") Integer size) { Page<CommentVO> commentVOPage = new Page<>(page, size); IPage<CommentVO> all = commentService.findAll(commentVOPage); return ApiResultHandler.successResult(all); }
这段代码看起来是一个 Spring Boot 的控制器,它定义了一个 GET 请求的路由 "/comments/{page}/{size}",其中 page 和 size 是路径参数,用于分页查询评论。控制器中通过调用 CommentService 的 findAll 方法来获取评论数据,并将其封装在一个 ApiResult 对象中返回给客户端。其中,ApiResultHandler 是一个用于处理 ApiResult 的工具类,它将数据转换成 JSON 格式的字符串并设置 HTTP 响应头信息。
@RestController @RequestMapping("/role") public class RoleController { @Resource private IRoleService roleService; // 新增或者更新 @PostMapping public Result save(@RequestBody Role role) { roleService.saveOrUpdate(role); return Result.success(); } @DeleteMapping("/{id}") public Result delete(@PathVariable Integer id) { roleService.removeById(id); return Result.success(); } @PostMapping("/del/batch") public Result deleteBatch(@RequestBody List<Integer> ids) { roleService.removeByIds(ids); return Result.success(); } @GetMapping public Result findAll() { return Result.success(roleService.list()); } @GetMapping("/{id}") public Result findOne(@PathVariable Integer id) { return Result.success(roleService.getById(id)); } @GetMapping("/page") public Result findPage(@RequestParam String name, @RequestParam Integer pageNum, @RequestParam Integer pageSize) { QueryWrapper<Role> queryWrapper = new QueryWrapper<>(); queryWrapper.like("name", name); queryWrapper.orderByDesc("id"); return Result.success(roleService.page(new Page<>(pageNum, pageSize), queryWrapper)); } /** * 绑定角色和菜单的关系 * @param roleId 角色id * @param menuIds 菜单id数组 * @return */ @PostMapping("/roleMenu/{roleId}") public Result roleMenu(@PathVariable Integer roleId, @RequestBody List<Integer> menuIds) { roleService.setRoleMenu(roleId, menuIds); return Result.success(); } @GetMapping("/roleMenu/{roleId}") public Result getRoleMenu(@PathVariable Integer roleId) { return Result.success( roleService.getRoleMenu(roleId)); } }
这是一个基于Spring Boot框架开发的角色管理控制器,提供了一些基本的角色管理操作,包括新增或更新角色、删除角色、批量删除角色、查询所有角色、分页查询角色和绑定角色和菜单的关系等操作。其中,IRoleService是角色管理服务接口,通过注入该接口的实现类,调用其中定义的方法来完成具体的业务逻辑处理。它采用的是RESTful风格的API设计,通过不同的HTTP方法(如POST、GET、DELETE等)和路径来区分不同的操作。
阅读全文
相关推荐
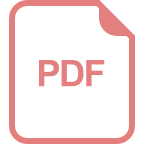













