idea中用mapper reduce提取一个文本文件数据,文件有价格,名字,厂商,等数据 分为价格5000及以上, 3000~5000, 3000以下,
时间: 2024-03-23 09:42:02 浏览: 45
首先,你需要定义一个Mapper函数来读取原始数据并将其转换为键值对的形式。假设你的数据格式为“价格,名字,厂商”,你可以使用逗号作为分隔符来将其拆分为三个字段。然后,你可以根据价格的大小将其分为三个范围,分别是“价格5000及以上”,“3000~5000”,“3000以下”。你可以使用以下代码来实现Mapper函数:
```java
public class PriceMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text priceRange = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
int price = Integer.parseInt(fields[0]);
if (price >= 5000) {
priceRange.set("价格5000及以上");
} else if (price >= 3000 && price < 5000) {
priceRange.set("3000~5000");
} else {
priceRange.set("3000以下");
}
context.write(priceRange, one);
}
}
```
接下来,你需要定义一个Reducer函数来对Mapper输出的键值对进行聚合并计算每个价格范围的数量。你可以使用以下代码来实现Reducer函数:
```java
public class PriceReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get();
}
context.write(key, new IntWritable(sum));
}
}
```
最后,你需要将Mapper和Reducer函数组合起来,并指定输入和输出路径来运行MapReduce程序。你可以使用以下代码来实现:
```java
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Price Count");
job.setJarByClass(PriceCount.class);
job.setMapperClass(PriceMapper.class);
job.setReducerClass(PriceReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
```
在运行程序时,你需要指定输入和输出路径,例如:
```sh
$ hadoop jar pricecount.jar /input/data.txt /output/pricecount
```
这将从`/input/data.txt`读取数据并将结果写入`/output/pricecount`。
阅读全文
相关推荐
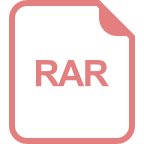
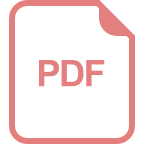


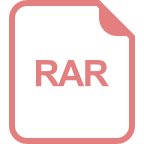
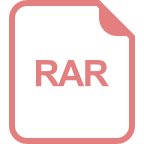
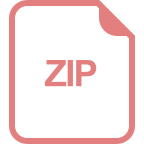
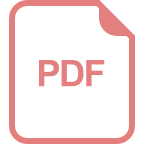
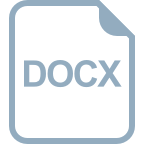
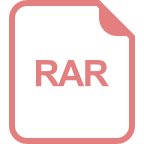
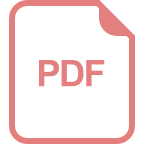

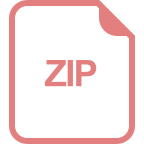
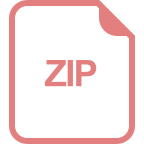