c语言把两个有序链表的合并的具体思路
时间: 2024-03-19 14:28:15 浏览: 30
C语言合并两个有序链表的具体思路如下:
1. 定义一个新的链表,用于存储合并后的结果
2. 分别定义两个指针,指向两个链表的头结点
3. 比较两个头结点的值,将较小的节点加入到新链表中
4. 移动较小节点所在链表的指针,继续与另一个链表头结点比较
5. 重复步骤3、4,直到一个链表没有元素
6. 将剩余链表的节点依次加入到新链表中
7. 返回新链表的头结点
注意:在链表节点数非常大时,需要考虑优化算法效率。可以采取快速排序等方法提高效率。
相关问题
c语言写两个有序链表合并
当我们需要合并两个有序链表时,我们可以使用迭代的方式来解决这个问题。首先,我们需要定义一个新的链表头节点,并设置一个指针用于遍历两个链表。然后,我们比较两个指针所指向的节点的值,将较小的节点添加到新链表中,并将指针向后移动。重复这个过程,直到其中一个链表遍历完毕。最后,将剩余链表的节点直接添加到新链表的末尾。
下面是使用C语言实现这个算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode* next;
};
// 创建新节点
struct ListNode* createNode(int val) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 合并两个有序链表
struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2) {
// 创建一个新的链表头节点
struct ListNode* dummy = createNode(0);
// 用于遍历新链表和两个原始链表
struct ListNode* cur = dummy;
while (l1 != NULL && l2 != NULL) {
// 比较两个节点的值
if (l1->val <= l2->val) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
// 将剩余链表的节点直接添加到新链表的末尾
if (l1 != NULL) {
cur->next = l1;
}
if (l2 != NULL) {
cur->next = l2;
}
return dummy->next;
}
// 打印链表
void printList(struct ListNode* head) {
struct ListNode* cur = head;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
// 创建两个有序链表
struct ListNode* l1 = createNode(1);
l1->next = createNode(3);
l1->next->next = createNode(5);
struct ListNode* l2 = createNode(2);
l2->next = createNode(4);
l2->next->next = createNode(6);
// 合并两个有序链表
struct ListNode* mergedList = mergeTwoLists(l1, l2);
// 打印合并后的链表
printf("Merged List: ");
printList(mergedList);
return 0;
}
```
运行上述代码,输出结果为:
```
Merged List: 1 2 3 4 5 6
```
这是将两个有序链表合并成一个有序链表的基本思路和实现方法。希望对你有帮助!
用c语言写两个有序链表合并成1个有序链表
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个新节点
Node* createNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 合并两个有序链表
Node* mergeLists(Node* l1, Node* l2) {
Node* head = createNode(0); // 创建一个头节点
Node* cur = head; // 当前节点指针
while (l1 && l2) {
if (l1->data <= l2->data) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
// 将未合并完的链表的剩余部分接到合并后的链表尾部
if (l1) {
cur->next = l1;
}
if (l2) {
cur->next = l2;
}
// 返回头节点的下一个节点
return head->next;
}
// 输出链表
void printList(Node* head) {
Node* cur = head;
while (cur) {
printf("%d ", cur->data);
cur = cur->next;
}
printf("\n");
}
int main() {
// 创建两个有序链表
Node* l1 = createNode(1);
l1->next = createNode(3);
l1->next->next = createNode(5);
Node* l2 = createNode(2);
l2->next = createNode(4);
l2->next->next = createNode(6);
// 合并两个有序链表
Node* mergedList = mergeLists(l1, l2);
// 输出合并后的链表
printList(mergedList);
return 0;
}
```
输出结果为:
```
1 2 3 4 5 6
```
相关推荐
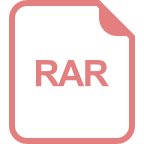












