protobuf base64编码, cpp列子
时间: 2023-08-29 16:05:47 浏览: 49
以下是一个简单的 C++ 列子,展示了如何使用 protobuf 库进行 base64 编码:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
#include <google/protobuf/message.h>
#include <google/protobuf/io/zero_copy_stream_impl_lite.h>
#include <google/protobuf/io/coded_stream.h>
#include <google/protobuf/util/base64.h>
using namespace std;
using namespace google::protobuf;
using namespace google::protobuf::io;
using namespace google::protobuf::util;
// 将 protobuf 消息进行 base64 编码
string protobufToBase64(const Message& message) {
string result;
string messageString;
// 将 protobuf 消息序列化为字符串
message.SerializeToString(&messageString);
// 对序列化后的字符串进行编码
Base64Encode(messageString, &result);
return result;
}
// 从 base64 编码中解码出 protobuf 消息
bool base64ToProtobuf(const string& base64String, Message& message) {
string decoded;
if (!Base64Decode(base64String, &decoded)) {
return false;
}
// 使用 ZeroCopyInputStream 和 CodedInputStream 解码出消息
const char* buffer = decoded.c_str();
int size = decoded.size();
ZeroCopyInputStream* input = new ArrayInputStream(buffer, size);
CodedInputStream* codedInput = new CodedInputStream(input);
codedInput->SetTotalBytesLimit(size, size);
bool success = message.ParseFromCodedStream(codedInput);
delete codedInput;
delete input;
return success;
}
int main() {
// 假设我们有一个 protobuf 消息 MyMessage,需要进行编码
MyMessage message;
message.set_id(123);
message.set_name("Hello World");
string encoded = protobufToBase64(message);
cout << "Encoded: " << encoded << endl;
// 假设我们已经从某个地方得到了 base64 编码,需要解码成 protobuf 消息
MyMessage decodedMessage;
bool success = base64ToProtobuf(encoded, decodedMessage);
if (success) {
cout << "Decoded ID: " << decodedMessage.id() << endl;
cout << "Decoded Name: " << decodedMessage.name() << endl;
} else {
cout << "Decoding failed." << endl;
}
return 0;
}
```
在该例子中,我们使用了 `google::protobuf::util::Base64Encode()` 和 `google::protobuf::util::Base64Decode()` 函数进行 base64 编码和解码。为了从 base64 编码中解码出 protobuf 消息,我们首先需要使用 `google::protobuf::util::Base64Decode()` 函数将 base64 编码解码成二进制数据,然后再使用 `google::protobuf::Message::ParseFromCodedStream()` 函数将二进制数据解析成 protobuf 消息。
相关推荐
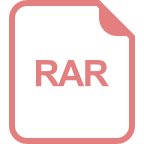














