用makefire编写可伸缩循环队列的静态库和动态库
时间: 2023-10-25 21:15:25 浏览: 89
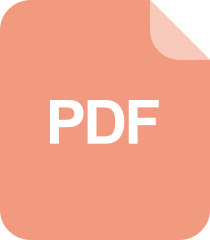
Eurofork伸缩货叉

下面给出一个示例,展示如何使用Makefile和Makefire编写可伸缩循环队列的静态库和动态库:
1. 创建工程目录和文件
```
$ mkdir queue
$ cd queue
$ touch queue.h queue.c main.c Makefile Makefire
```
2. 编写queue.h和queue.c文件
queue.h文件:
```c
#ifndef QUEUE_H
#define QUEUE_H
typedef struct {
int *data;
int head;
int tail;
int size;
} Queue;
Queue *queue_create(int size);
void queue_destroy(Queue *q);
void queue_push(Queue *q, int value);
int queue_pop(Queue *q);
int queue_size(Queue *q);
#endif
```
queue.c文件:
```c
#include "queue.h"
#include <stdlib.h>
Queue *queue_create(int size) {
Queue *q = malloc(sizeof(Queue));
q->data = malloc(sizeof(int) * size);
q->head = 0;
q->tail = 0;
q->size = size;
return q;
}
void queue_destroy(Queue *q) {
free(q->data);
free(q);
}
void queue_push(Queue *q, int value) {
q->data[q->tail] = value;
q->tail = (q->tail + 1) % q->size;
}
int queue_pop(Queue *q) {
int value = q->data[q->head];
q->head = (q->head + 1) % q->size;
return value;
}
int queue_size(Queue *q) {
if (q->head <= q->tail) {
return q->tail - q->head;
} else {
return q->tail + q->size - q->head;
}
}
```
3. 编写main.c文件
```c
#include "queue.h"
#include <stdio.h>
int main() {
Queue *q = queue_create(5);
queue_push(q, 1);
queue_push(q, 2);
queue_push(q, 3);
queue_push(q, 4);
queue_push(q, 5);
printf("size: %d\n", queue_size(q));
printf("pop: %d\n", queue_pop(q));
printf("pop: %d\n", queue_pop(q));
printf("size: %d\n", queue_size(q));
queue_push(q, 6);
queue_push(q, 7);
printf("size: %d\n", queue_size(q));
queue_destroy(q);
return 0;
}
```
4. 编写Makefile文件
```makefile
CC = gcc
CFLAGS = -Wall -g
LDFLAGS_STATIC = -static
LDFLAGS_DYNAMIC = -shared
SRC = queue.c main.c
OBJ = $(SRC:.c=.o)
TARGET_STATIC = libqueue.a
TARGET_DYNAMIC = libqueue.so
all: $(TARGET_STATIC) $(TARGET_DYNAMIC)
$(TARGET_STATIC): $(OBJ)
ar rcs $@ $^
$(TARGET_DYNAMIC): $(OBJ)
$(CC) $(LDFLAGS_DYNAMIC) -o $@ $^
%.o: %.c
$(CC) $(CFLAGS) -c -o $@ $<
clean:
rm -f $(OBJ) $(TARGET_STATIC) $(TARGET_DYNAMIC)
```
5. 编写Makefire文件
```
BUILD_DIR := build
LIB_DIR := lib
INCLUDE_DIR := include
SRC_DIR := .
CFLAGS := -Wall -g
LDFLAGS :=
TARGET := queue
STATIC_LIB := $(LIB_DIR)/$(TARGET).a
DYNAMIC_LIB := $(LIB_DIR)/$(TARGET).so
HEADER := $(INCLUDE_DIR)/$(TARGET).h
SRC_FILES := $(wildcard $(SRC_DIR)/*.c)
OBJ_FILES := $(patsubst $(SRC_DIR)/%.c,$(BUILD_DIR)/%.o,$(SRC_FILES))
all: static dynamic
static: $(STATIC_LIB)
dynamic: $(DYNAMIC_LIB)
$(STATIC_LIB): $(OBJ_FILES)
ar rcs $@ $^
$(DYNAMIC_LIB): $(OBJ_FILES)
$(CC) $(LDFLAGS) -shared -o $@ $^
$(BUILD_DIR)/%.o: $(SRC_DIR)/%.c
mkdir -p $(BUILD_DIR)
$(CC) $(CFLAGS) -c -fPIC -o $@ $<
install: $(HEADER) $(STATIC_LIB) $(DYNAMIC_LIB)
mkdir -p /usr/local/include/$(TARGET)
cp $(HEADER) /usr/local/include/$(TARGET)
cp $(STATIC_LIB) /usr/local/lib
cp $(DYNAMIC_LIB) /usr/local/lib
uninstall:
rm -rf /usr/local/include/$(TARGET)
rm -f /usr/local/lib/$(STATIC_LIB)
rm -f /usr/local/lib/$(DYNAMIC_LIB)
clean:
rm -rf $(BUILD_DIR) $(LIB_DIR)/*.a $(LIB_DIR)/*.so
```
6. 在终端中运行make命令或makefire命令编译程序
```
$ make
$ makefire
```
编译完成后,生成的静态库和动态库文件分别为:
- libqueue.a
- libqueue.so
可执行文件为:
- main
该示例程序演示了如何使用Makefile和Makefire编写可伸缩循环队列的静态库和动态库。需要注意的是,Makefile和Makefire文件中的编译选项可能需要根据不同的系统和编译器进行调整。
阅读全文
相关推荐
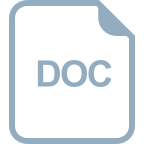
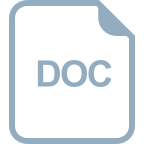








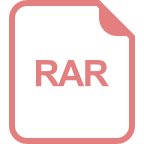
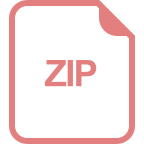
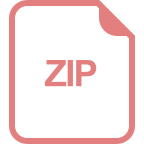
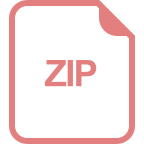
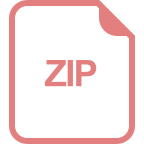
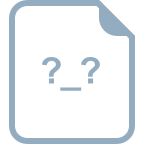
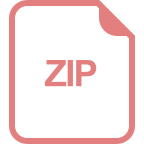
