用C语言设计一套线程池接口(不用实现),要求该接口可以完成线程池创建、销毁、以及传入某个回调函数并在线程中执行的功能。
时间: 2024-03-18 14:38:35 浏览: 18
好的,根据您的需求,我可以提供以下用C语言设计的线程池接口:
```c
typedef struct threadpool_t threadpool_t;
// 线程池中执行的任务函数类型
typedef void (*threadpool_task_func_t)(void *);
// 创建线程池
threadpool_t *threadpool_create(int thread_count);
// 销毁线程池
void threadpool_destroy(threadpool_t *pool);
// 添加任务到线程池
void threadpool_add_task(threadpool_t *pool, threadpool_task_func_t func, void *arg);
// 等待线程池中所有任务执行完毕
void threadpool_wait(threadpool_t *pool);
// 阻塞地获取线程池中的任务,并执行该任务
void threadpool_get_task(threadpool_t *pool);
// 停止线程池
void threadpool_stop(threadpool_t *pool);
```
通过上述接口,我们可以完成线程池的创建、销毁以及传入某个回调函数并在线程中执行的功能。具体实现细节可以根据具体需求进行调整。
相关问题
用C语言写一个动态创建与销毁线程池中线程的函数
可以使用pthread_create和pthread_join函数来动态创建和销毁线程池中的线程。具体示例代码如下:// 动态创建线程
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg);// 销毁线程
int pthread_join(pthread_t thread, void **retval);
回调函数如何在c语言中实现
在C语言中,回调函数是通过函数指针来实现的。回调函数本质上是一个普通的函数,但是它会被另一个函数作为参数传递,并且在需要的时候被调用执行。
以下是在C语言中实现回调函数的步骤:
1. 首先定义一个回调函数的函数指针类型,这个类型与回调函数的参数列表和返回值类型相匹配。
例如,如果回调函数的参数列表为 int 和 float,则函数指针类型可以定义为:
```
typedef void (*CallbackFunc)(int, float);
```
2. 在需要使用回调函数的函数中,定义一个函数指针变量,用来存储回调函数的地址。
例如,如果需要在某个函数中调用回调函数,则可以定义一个函数指针变量:
```
CallbackFunc callback;
```
3. 在函数中调用回调函数。
例如,如果需要在某个函数中调用回调函数,则可以使用以下代码:
```
if (callback != NULL) {
callback(10, 3.14);
}
```
4. 在调用函数的地方,将实际的回调函数作为参数传递给函数。
例如,如果需要将名为 myCallback 的函数作为回调函数传递给函数,则可以使用以下代码:
```
void myCallback(int a, float b) {
printf("a = %d, b = %f\n", a, b);
}
// ...
callback = myCallback;
```
这样,在调用函数时,实际的回调函数 myCallback 就会被调用并执行相应的操作。
相关推荐
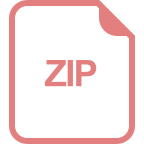
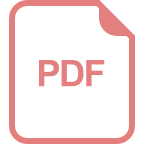












