用c#定义一个抽象类Person,其包含抽象的Introduce方法。定义一个Student类,继承Person类,并实现Introduce方法。
时间: 2024-10-24 17:18:18 浏览: 33
在C#中,你可以定义一个抽象类`Person`,其中包含一个抽象方法`Introduce`,就像这样:
```csharp
using System;
// 抽象类Person
public abstract class Person
{
// 抽象的Introduce方法,没有具体的实现
public abstract void Introduce();
}
// 子类Student继承自Person,并实现Introduce方法
public class Student : Person
{
// 在Student类中提供Introduce方法的具体实现
public override void Introduce()
{
Console.WriteLine("我是学生,正在自我介绍...");
}
}
```
在这个例子中,`Person`是一个抽象类,因为它的`Introduce`方法没有具体实现,所以必须在任何子类中被覆盖。`Student`类继承了`Person`并实现了`Introduce`方法,这意味着当你创建`Student`对象并调用`Introduce`方法时,会执行`Student`版本的实现。
注意,如果你尝试直接创建一个`Person`对象,而不通过`Student`或其他实现`Introduce`方法的子类,将会得到编译错误,因为`Person`是抽象的,不能直接实例化。
相关问题
用c# 定义一个抽象类Person,其包含抽象的Introduce方法。定义一个Student类,继承Person类,并实现Introduce方法。
在 C# 中,你可以定义一个抽象类 `Person` 作为基础类,其中包含一个抽象方法 `Introduce`,表示这个方法需要在其派生类中被具体实现。下面是具体的代码示例:
```csharp
using System;
// 抽象类 Person
public abstract class Person
{
// 抽象方法 Introduce
public abstract void Introduce();
}
// 子类 Student 继承自 Person 并实现 Introduce 方法
public class Student : Person
{
// 实现 Introduce 方法的具体内容
public override void Introduce()
{
Console.WriteLine("我是学生,正在学习中...");
}
}
```
在这个例子中,`Student` 类通过 `override` 关键字实现了从 `Person` 类继承来的抽象方法 `Introduce`。当你创建 `Student` 的实例并调用 `Introduce` 时,会打印出 "我是学生,正在学习中..." 这样的文本。
用C#定义一个抽象类Person,其包含抽象的Introduce方法。定义一个Student类,继承Person类,并实现Introduce方法。
在C#中,你可以通过以下步骤定义一个抽象类`Person`并实现抽象方法`Introduce`:
```csharp
// 定义抽象类 Person
public abstract class Person
{
// 抽象方法 Introduce
public abstract void Introduce();
}
// 子类 Student 继承 Person 并实现 Introduce 方法
public class Student : Person
{
// 实现 Introduce 方法的具体内容
public override void Introduce()
{
Console.WriteLine("我是学生,正在学习中...");
}
}
```
在这个例子中,`Person`是一个抽象类,它有一个抽象方法`Introduce`,这意味着任何直接从`Person`派生的类都必须提供对这个方法的具体实现。而`Student`类正是这样一个继承了`Person`的类,并实现了`Introduce`方法,输出特定的信息。
阅读全文
相关推荐
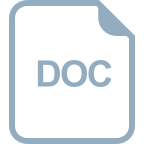
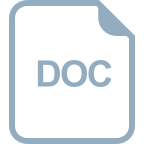
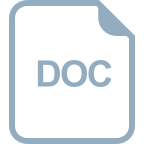













