某大学有n个宿舍楼,要求用c语言实现n个宿舍楼之间的电网能够互相接通,构造宿舍楼之间的电网,使总工程造价最低。(1)建立n个宿舍楼的带权无向图(宿舍楼表示顶点,宿舍楼距离为权值) 至少完成以下功能: (2)采用克鲁斯卡尔算法构造最小生成树 (3)算出工程造价。
时间: 2024-04-09 19:28:03 浏览: 101
你可以使用以下代码来实现这个问题:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义边的结构体
typedef struct Edge {
int src, dest, weight;
} Edge;
// 定义图的结构体
typedef struct Graph {
int V, E;
Edge* edges;
} Graph;
// 创建图
Graph* createGraph(int V, int E) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->V = V;
graph->E = E;
graph->edges = (Edge*)malloc(E * sizeof(Edge));
return graph;
}
// 查找父节点
int findParent(int parent[], int i) {
if (parent[i] == -1)
return i;
return findParent(parent, parent[i]);
}
// 合并两个集合
void unionSet(int parent[], int x, int y) {
int xset = findParent(parent, x);
int yset = findParent(parent, y);
parent[xset] = yset;
}
// 比较两个边的权值,用于排序
int compare(const void* a, const void* b) {
Edge* a1 = (Edge*)a;
Edge* b1 = (Edge*)b;
return a1->weight - b1->weight;
}
// 构造最小生成树
void constructMST(Graph* graph) {
int V = graph->V;
Edge result[V];
int e = 0;
int i = 0;
qsort(graph->edges, graph->E, sizeof(graph->edges[0]), compare);
int parent[V];
for (int v = 0; v < V; v++)
parent[v] = -1;
while (e < V - 1 && i < graph->E) {
Edge next_edge = graph->edges[i++];
int x = findParent(parent, next_edge.src);
int y = findParent(parent, next_edge.dest);
if (x != y) {
result[e++] = next_edge;
unionSet(parent, x, y);
}
}
int total_cost = 0;
for (i = 0; i < e; i++) {
printf("%d -- %d == %d\n", result[i].src, result[i].dest, result[i].weight);
total_cost += result[i].weight;
}
printf("工程造价: %d\n", total_cost);
}
int main() {
int n, E;
printf("请输入宿舍楼的个数: ");
scanf("%d", &n);
E = n * (n - 1) / 2; // 完全图的边数
Graph* graph = createGraph(n, E);
int k = 0;
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
int weight;
printf("请输入宿舍楼 %d 到宿舍楼 %d 的距离: ", i, j);
scanf("%d", &weight);
graph->edges[k].src = i;
graph->edges[k].dest = j;
graph->edges[k].weight = weight;
k++;
}
}
constructMST(graph);
return 0;
}
```
这段代码使用了克鲁斯卡尔算法来构造最小生成树,并计算了工程造价。你可以根据提示输入宿舍楼之间的距离,程序将输出构造的电网和总工程造价。
阅读全文
相关推荐
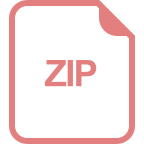
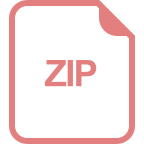
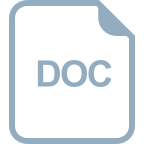
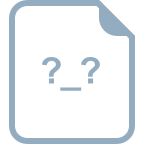
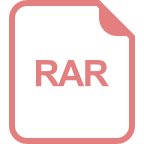
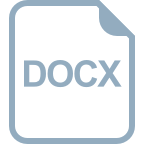
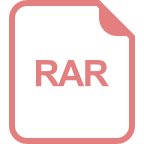
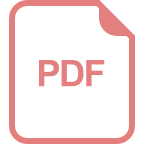
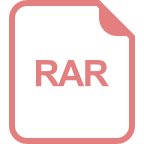
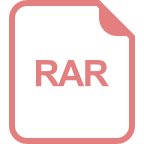
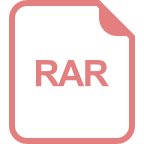