c语言求二叉树节点的最大值
时间: 2023-07-13 07:19:36 浏览: 195
以下是求二叉树节点最大值的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct node {
int value;
struct node *left;
struct node *right;
} node;
// 创建一个新的节点
node *new_node(int value) {
node *n = (node *) malloc(sizeof(node));
n->value = value;
n->left = NULL;
n->right = NULL;
return n;
}
// 按照给定的数值插入到二叉树中
void insert(node **root, int value) {
if (*root == NULL) {
*root = new_node(value);
return;
}
if (value < (*root)->value) {
insert(&(*root)->left, value);
} else {
insert(&(*root)->right, value);
}
}
// 递归求二叉树节点最大值
int max_value(node *root) {
if (root == NULL) {
return -1;
} else if (root->right == NULL) {
return root->value;
} else {
return max_value(root->right);
}
}
int main() {
node *root = NULL;
insert(&root, 5);
insert(&root, 3);
insert(&root, 7);
insert(&root, 1);
insert(&root, 9);
printf("最大值为:%d\n", max_value(root));
return 0;
}
```
以上代码中,通过递归求出二叉树中右子树最底层节点的值,即为二叉树节点的最大值。
阅读全文
相关推荐


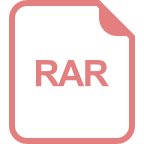














