用C语言编写编写计算二叉树最大宽度的算法。(二叉树最大宽度指二叉树所有层中结点个数的最大值)
时间: 2024-02-06 19:11:20 浏览: 118
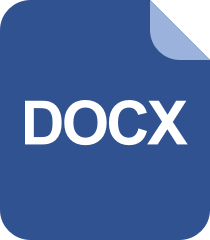
数据结构:二叉树层次遍历算法解析及C语言实现
可以使用队列来实现对二叉树的层序遍历,统计每层的结点个数,最后求出最大值即可。
以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树结点结构体
typedef struct node {
int data;
struct node *left;
struct node *right;
} Node;
// 队列结构体
typedef struct queue {
Node **data;
int front;
int rear;
} Queue;
// 初始化队列
Queue *initQueue(int size) {
Queue *q = (Queue *)malloc(sizeof(Queue));
q->data = (Node **)malloc(sizeof(Node *) * size);
q->front = q->rear = 0;
return q;
}
// 入队
void enQueue(Queue *q, Node *node) {
q->data[q->rear++] = node;
}
// 出队
Node *deQueue(Queue *q) {
return q->data[q->front++];
}
// 判断队列是否为空
int isQueueEmpty(Queue *q) {
return q->front == q->rear;
}
// 计算二叉树最大宽度
int maxWidth(Node *root) {
if (root == NULL) {
return 0;
}
Queue *q = initQueue(100);
enQueue(q, root);
int width = 1;
while (!isQueueEmpty(q)) {
int size = q->rear - q->front;
if (size > width) {
width = size;
}
for (int i = 0; i < size; i++) {
Node *cur = deQueue(q);
if (cur->left != NULL) {
enQueue(q, cur->left);
}
if (cur->right != NULL) {
enQueue(q, cur->right);
}
}
}
return width;
}
int main() {
// 构造如下二叉树
// 1
// / \
// 2 3
// / \ \
// 4 5 6
Node *root = (Node *)malloc(sizeof(Node));
root->data = 1;
root->left = (Node *)malloc(sizeof(Node));
root->left->data = 2;
root->left->left = (Node *)malloc(sizeof(Node));
root->left->left->data = 4;
root->left->right = (Node *)malloc(sizeof(Node));
root->left->right->data = 5;
root->right = (Node *)malloc(sizeof(Node));
root->right->data = 3;
root->right->right = (Node *)malloc(sizeof(Node));
root->right->right->data = 6;
printf("二叉树最大宽度为:%d\n", maxWidth(root)); // 输出:二叉树最大宽度为:3
return 0;
}
```
注:代码中使用了队列的数据结构,需要先实现队列的初始化、入队、出队、判断队列是否为空等操作。
阅读全文
相关推荐
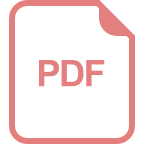
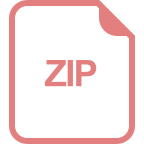















