7. 编写递归算法:求二叉树中以元素值为x的结点为根的子树的深度。用C语言写
时间: 2024-12-22 07:13:32 浏览: 14
在C语言中,编写一个递归算法来计算二叉树中以给定值 `x` 的节点作为根的子树的深度,可以按照以下步骤进行:
```c
#include <stdio.h>
// 定义二叉树结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 计算以val为根的子树深度函数
int depthOfSubtree(TreeNode* root, int x) {
// 如果root为空,返回0表示深度为0
if (root == NULL) {
return 0;
}
// 如果当前节点值等于目标值x,递归查找左、右子树的最大深度
if (root->val == x) {
int leftDepth = depthOfSubtree(root->left, x);
int rightDepth = depthOfSubtree(root->right, x);
// 返回左右子树深度较大者加1(因为根节点也算一层)
return (leftDepth > rightDepth ? leftDepth : rightDepth) + 1;
} else {
// 如果当前节点值不等于x,直接递归查找左右子树
return 1 + max(depthOfSubtree(root->left, x), depthOfSubtree(root->right, x));
}
}
// 辅助函数计算最大值
int max(int a, int b) {
return a > b ? a : b;
}
// 主函数测试
int main() {
// 假设已经有一个二叉树实例,例如:
// 5
// / \
// 3 8
// / \
// 2 4
// 其中根节点值为5
TreeNode* root = ...; // 实际填充二叉树的节点
int x = 5; // 搜索的目标值
int result = depthOfSubtree(root, x);
printf("子树深度: %d\n", result);
return 0;
}
```
这个算法通过递归遍历二叉树,对于每个节点检查其值是否等于目标值 `x`,如果等于则继续在左右子树上查找,直到找到所有以 `x` 为根的子树,并返回其中最深的那个子树的深度。
阅读全文
相关推荐
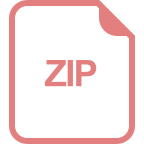
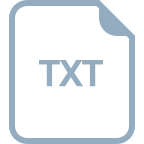
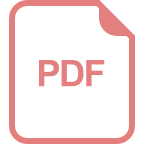
















