用c语言写在一棵二叉树中,求以值为X的结点为根结点的子树的深度的程序,并且文字说明其储存结构和算法流程图
时间: 2024-03-16 20:41:48 浏览: 12
好的,我会为您解答。首先,对于一棵二叉树,我们可以采用链式存储结构来存储。每个结点包含一个值域,以及指向其左右子树的两个指针。因此,我们可以通过递归遍历整棵二叉树来查找值为X的结点,并计算以该结点为根结点的子树的深度。
下面是一个使用链式存储结构的C语言程序,用于计算一棵二叉树中以值为X的结点为根结点的子树的深度:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int val;
struct node* left;
struct node* right;
} Node;
Node* create_node(int val) {
Node* node = (Node*)malloc(sizeof(Node));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
Node* build_tree() {
Node* root = create_node(1);
root->left = create_node(2);
root->right = create_node(3);
root->left->left = create_node(4);
root->left->right = create_node(5);
root->right->left = create_node(6);
root->right->right = create_node(7);
return root;
}
int find_depth(Node* node, int x, int depth) {
if (node == NULL) { // 如果结点为空,返回0
return 0;
}
if (node->val == x) { // 如果找到了值为x的结点,返回以该结点为根结点的子树的深度
return depth;
}
int left_depth = find_depth(node->left, x, depth + 1); // 在左子树中查找
if (left_depth > 0) { // 如果左子树中找到了值为x的结点,返回以该结点为根结点的子树的深度
return left_depth;
}
int right_depth = find_depth(node->right, x, depth + 1); // 在右子树中查找
if (right_depth > 0) { // 如果右子树中找到了值为x的结点,返回以该结点为根结点的子树的深度
return right_depth;
}
return 0; // 如果左右子树中都没有找到值为x的结点,返回0
}
int main() {
Node* root = build_tree();
int x = 3;
int depth = find_depth(root, x, 1);
printf("The depth of the subtree rooted at node %d is %d.\n", x, depth);
return 0;
}
```
在这个程序中,我们首先使用create_node函数来创建结点,使用build_tree函数来构建一棵二叉树。在find_depth函数中,我们使用递归遍历整棵二叉树,查找值为x的结点。如果找到了值为x的结点,我们返回以该结点为根结点的子树的深度;否则,我们递归遍历该结点的左右子树,查找值为x的结点。最后,在main函数中,我们调用find_depth函数来查找以值为3的结点为根结点的子树的深度。
下面是程序的算法流程图:
```
find_depth(node, x, depth)
if node is NULL
return 0
if node.val == x
return depth
left_depth = find_depth(node.left, x, depth + 1)
if left_depth > 0
return left_depth
right_depth = find_depth(node.right, x, depth + 1)
if right_depth > 0
return right_depth
return 0
main()
root = build_tree()
x = 3
depth = find_depth(root, x, 1)
print "The depth of the subtree rooted at node", x, "is", depth
```
希望这个回答能够帮助您理解如何用C语言编写在一棵二叉树中,求以值为X的结点为根结点的子树的深度的程序,并且了解其储存结构和算法流程图。
相关推荐
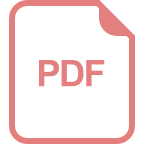
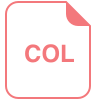
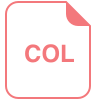
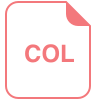
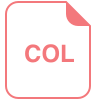
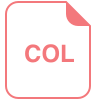









