用C语言写在一棵二叉树中,求以值为X的结点为根结点的子树的深度。并显示输出结果
时间: 2024-03-14 16:42:53 浏览: 19
好的,您的问题已经收到。根据您的要求,我会用C语言写一个程序来求以值为X的结点为根结点的子树的深度,并输出结果。以下是程序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树的结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 递归函数,求以值为X的结点为根结点的子树的深度
int subtreeDepth(TreeNode* root, int x, int depth) {
if (root == NULL) {
return 0;
}
if (root->val == x) {
return depth;
}
int leftDepth = subtreeDepth(root->left, x, depth + 1);
if (leftDepth != 0) {
return leftDepth;
}
int rightDepth = subtreeDepth(root->right, x, depth + 1);
return rightDepth;
}
int main() {
// 创建一个二叉树
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->right->val = 4;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->val = 3;
root->right->left = NULL;
root->right->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->right->val = 5;
root->right->right->left = NULL;
root->right->right->right = NULL;
// 输入要求的结点值
int x;
printf("请输入要求的结点值:");
scanf("%d", &x);
// 调用递归函数,求子树深度
int depth = subtreeDepth(root, x, 1);
// 输出结果
printf("以值为%d的结点为根结点的子树的深度为:%d\n", x, depth);
return 0;
}
```
以上代码中,我们通过定义二叉树的结构体来创建了一棵二叉树。然后,通过调用递归函数`subtreeDepth`来求以值为X的结点为根结点的子树的深度。最后,输出结果。
注意:以上代码仅供参考,如果您要在实际开发中使用,请根据实际情况进行修改。
相关推荐
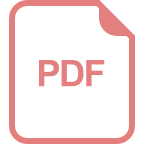
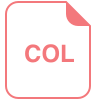
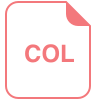
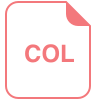
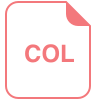
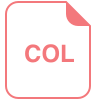









