用c语言求在一颗二叉树中,求以值为x的结点为根节点的子树深度
时间: 2024-03-14 14:43:13 浏览: 77
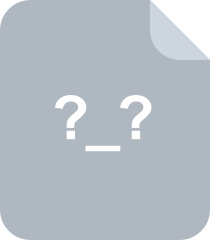
求二叉树的深度

好的,我可以为您提供一个二叉树的深度优先遍历的递归算法,用于搜索并计算以值为x的节点为根节点的子树深度。
首先,我们定义一个二叉树的节点结构体,它包含一个整型值val,以及两个指向左右子节点的指针left和right。
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
```
然后,我们定义一个递归函数`getDepth`,它的参数为当前节点root和目标值x,返回值为以当前节点为根节点的子树深度。
```c
int getDepth(struct TreeNode* root, int x) {
// 如果当前节点为空,返回0
if (!root) return 0;
// 如果当前节点的值等于x,就以它为根节点计算子树深度
if (root->val == x) {
// 子树深度 = max(左子树深度, 右子树深度) + 1
return max(getDepth(root->left, x), getDepth(root->right, x)) + 1;
}
// 否则递归搜索左右子树
return max(getDepth(root->left, x), getDepth(root->right, x));
}
```
最后,我们可以在主函数中创建一颗二叉树,并调用`getDepth`函数计算以值为x的节点为根节点的子树深度。
```c
int main() {
// 创建一颗二叉树
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->right->val = 3;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->val = 4;
root->right->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->left->val = 5;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->right->val = 6;
root->right->right->left = NULL;
root->right->right->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->right->right->val = 7;
root->right->right->right->left = NULL;
root->right->right->right->right = NULL;
// 计算以值为x的节点为根节点的子树深度
int x = 4;
int depth = getDepth(root, x);
// 输出结果
printf("以值为%d的节点为根节点的子树深度为%d\n", x, depth);
return 0;
}
```
注意,这里我们使用了一个辅助函数`max`来比较两个数的大小,它的实现如下:
```c
int max(int a, int b) {
return a > b ? a : b;
}
```
希望这个算法能够对您有所帮助!
阅读全文
相关推荐
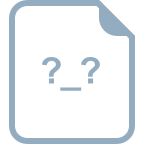














