写出C语言代码在二叉树查找是否存在值为x的结点,其中二叉树需要先序递归输入
时间: 2023-06-10 20:03:48 浏览: 97
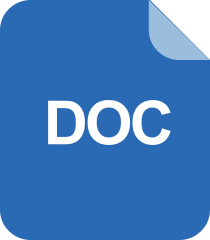
C语言 求一个二叉树中位于先序序列中第k个位置的结点的值.doc
好的,以下是根据您的要求编写的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
/* 定义二叉树结构体 */
typedef struct Node {
int data;
struct Node *left;
struct Node *right;
} Node;
/* 先序递归输入二叉树节点 */
void createBinaryTree(Node **root) {
int x;
scanf("%d", &x);
if (x == -1) {
*root = NULL;
} else {
*root = (Node*) malloc(sizeof(Node));
(*root)->data = x;
createBinaryTree(&((*root)->left));
createBinaryTree(&((*root)->right));
}
}
/* 二叉树查找 */
Node *search(Node *root, int x) {
if (root == NULL) {
return NULL;
}
if (root->data == x) {
return root;
}
Node *leftResult = search(root->left, x);
if (leftResult != NULL) {
return leftResult;
}
Node *rightResult = search(root->right, x);
if (rightResult != NULL) {
return rightResult;
}
return NULL;
}
/* 主函数 */
int main() {
Node *root;
printf("请输入先序递归序列(-1表示空节点):\n");
createBinaryTree(&root);
int x;
printf("请输入要查找的节点值x:");
scanf("%d", &x);
Node *result = search(root, x);
if (result != NULL) {
printf("找到了节点值为%d的节点!", x);
} else {
printf("未找到节点值为%d的节点!", x);
}
return 0;
}
```
希望能够帮助到您!
阅读全文
相关推荐
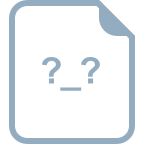
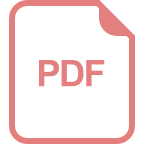













