给出使用C语言代码,用于实现二叉树的二叉链表储存、二叉树的先序/中序/后序递归遍历、统计二叉树的高度、统计结点的个数、叶子结点的个数、先序/中序非递归遍历、层序遍历
时间: 2023-06-16 14:05:10 浏览: 68
二叉树的二叉链表储存:
```
typedef struct BiTNode{
int data;
struct BiTNode *lchild, *rchild;
}BiTNode, *BiTree;
```
先序/中序/后序递归遍历:
```
void PreOrderTraverse(BiTree T){
if(T==NULL){
return;
}
printf("%d ", T->data);
PreOrderTraverse(T->lchild);
PreOrderTraverse(T->rchild);
}
void InOrderTraverse(BiTree T){
if(T==NULL){
return;
}
InOrderTraverse(T->lchild);
printf("%d ", T->data);
InOrderTraverse(T->rchild);
}
void PostOrderTraverse(BiTree T){
if(T==NULL){
return;
}
PostOrderTraverse(T->lchild);
PostOrderTraverse(T->rchild);
printf("%d ", T->data);
}
```
统计二叉树的高度:
```
int GetHeight(BiTree T){
if(T==NULL){
return 0;
}
int left_height = GetHeight(T->lchild);
int right_height = GetHeight(T->rchild);
return left_height > right_height ? left_height+1 : right_height+1;
}
```
统计结点的个数:
```
int GetNodeCount(BiTree T){
if(T==NULL){
return 0;
}
int left_count = GetNodeCount(T->lchild);
int right_count = GetNodeCount(T->rchild);
return left_count + right_count + 1;
}
```
叶子结点的个数:
```
int GetLeafCount(BiTree T){
if(T==NULL){
return 0;
}
if(T->lchild==NULL && T->rchild==NULL){
return 1;
}
int left_count = GetLeafCount(T->lchild);
int right_count = GetLeafCount(T->rchild);
return left_count + right_count;
}
```
先序/中序非递归遍历:
```
void PreOrder(BiTree T){
if(T==NULL){
return;
}
BiTree stack[100];
int top = -1;
stack[++top] = T;
while(top!=-1){
BiTree node = stack[top--];
printf("%d ", node->data);
if(node->rchild!=NULL){
stack[++top] = node->rchild;
}
if(node->lchild!=NULL){
stack[++top] = node->lchild;
}
}
}
void InOrder(BiTree T){
if(T==NULL){
return;
}
BiTree stack[100];
int top = -1;
BiTree node = T;
while(top!=-1 || node!=NULL){
while(node!=NULL){
stack[++top] = node;
node = node->lchild;
}
node = stack[top--];
printf("%d ", node->data);
node = node->rchild;
}
}
```
层序遍历:
```
void LevelOrder(BiTree T){
if(T==NULL){
return;
}
BiTree queue[100];
int front = 0;
int rear = 0;
queue[rear++] = T;
while(front!=rear){
BiTree node = queue[front++];
printf("%d ", node->data);
if(node->lchild!=NULL){
queue[rear++] = node->lchild;
}
if(node->rchild!=NULL){
queue[rear++] = node->rchild;
}
}
}
```
相关推荐
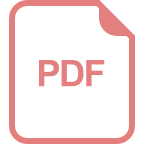
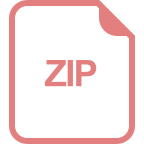
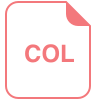
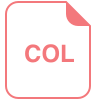
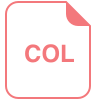
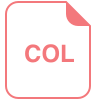
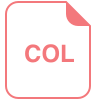









