用c语言1.编写函数,输入字符序列,建立二叉树的二叉链表 2.编写函数,实现二叉树的中序递归遍历算法。 3.编写函数,实现二叉树的中序非递归遍历算法 4.编写函数,借助队列实现二叉树的层次遍历算法 5.编写函数,求二叉树的高度 6.编写函数,求二叉树的结点个数 7.编写函数,求二叉树的叶子个数 8.编写函数,交换二叉树每个结点的左子树和右子树编写一个主函数,在主函数中设计一个简单的菜单,
时间: 2023-06-03 20:08:05 浏览: 87
1. 很好,我可以为您提供一些关于二叉树的算法问题的解答。关于您的第一个问题,以下是使用C语言建立二叉树的二叉链表的函数:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *left;
struct node *right;
} Node;
Node* create_tree() {
char ch;
Node *p;
scanf("%c", &ch);
if (ch == '#') {
p = NULL;
} else {
p = (Node*)malloc(sizeof(Node));
p->data = ch;
p->left = create_tree();
p->right = create_tree();
}
return p;
}
int main() {
Node* root = create_tree();
return 0;
}
```
2. 接下来,这是使用递归算法实现二叉树的中序遍历的函数:
```c
void inorder_traversal(Node *root) {
if (root == NULL) {
return;
}
inorder_traversal(root->left);
printf("%c ", root->data);
inorder_traversal(root->right);
}
```
3. 这是使用非递归算法实现二叉树的中序遍历的函数:
```c
void inorder_traversal_no_recursive(Node *root) {
if (root == NULL) {
return;
}
Node *stack[100];
int top = -1;
Node *cur = root;
while (cur != NULL || top >= 0) {
while (cur != NULL) {
stack[++top] = cur;
cur = cur->left;
}
cur = stack[top--];
printf("%c ", cur->data);
cur = cur->right;
}
}
```
4. 借助队列实现二叉树的层次遍历算法,以下是对应的函数:
```c
void level_traversal(Node *root) {
if (root == NULL) {
return;
}
Node *queue[100];
int front = 0, rear = 0;
queue[rear++] = root;
while (front != rear) {
Node *cur = queue[front++];
printf("%c ", cur->data);
if (cur->left != NULL) {
queue[rear++] = cur->left;
}
if (cur->right != NULL) {
queue[rear++] = cur->right;
}
}
}
```
5. 这是求解二叉树高度的函数:
```c
int tree_height(Node *root) {
if (root == NULL) {
return 0;
}
int left_height = tree_height(root->left);
int right_height = tree_height(root->right);
return left_height > right_height ? left_height + 1 : right_height + 1;
}
```
6. 下面是求解二叉树结点个数的函数:
```c
int node_count(Node *root) {
if (root == NULL) {
return 0;
}
return node_count(root->left) + node_count(root->right) + 1;
}
```
7. 关于求解二叉树叶子结点个数的函数,这是对应的实现:
```c
int leaf_count(Node *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return leaf_count(root->left) + leaf_count(root->right);
}
```
8. 最后,这是交换二叉树每个节点的左子树和右子树的函数:
```c
void swap_tree(Node *root) {
if (root == NULL) {
return;
}
Node *tmp = root->left;
root->left = root->right;
root->right = tmp;
swap_tree(root->left);
swap_tree(root->right);
}
```
在主函数中,您可以使用C语言的基本输入输出函数编写一个交互式菜单,便于用户选择需要执行的子函数。
相关推荐
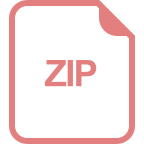
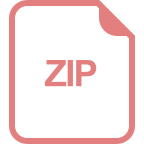












