C语言或C++用递归算法求取二叉树每层结点的个数,从而求取二叉树的宽度
时间: 2023-05-26 11:04:06 浏览: 171
递归算法实现二叉树每层结点个数和宽度的思路如下:
1. 定义一个函数计算二叉树某一层的结点个数,该函数需要传入二叉树的根结点指针、层数和一个计数器。在函数中,如果当前的层数等于1,则将计数器加1;否则递归调用该函数计算左子树和右子树在下一层的结点个数,并将计数器累加上这两个返回值。
2. 定义另一个函数计算二叉树的宽度,该函数需要传入二叉树的根结点指针和二叉树的深度。在函数中,循环调用第一步定义的函数,分别计算每一层的结点个数,并将这些个数求最大值,即为二叉树的宽度。
以下是详细的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
int countLevelNodes(TreeNode *root, int level, int count) {
if (!root) {
return count;
}
if (level == 1) {
return count + 1;
}
count = countLevelNodes(root->left, level - 1, count);
count = countLevelNodes(root->right, level - 1, count);
return count;
}
int getWidth(TreeNode *root, int depth) {
int width = 0, i, count;
for (i = 1; i <= depth; i++) {
count = countLevelNodes(root, i, 0);
if (count > width) {
width = count;
}
}
return width;
}
int main() {
/* 构造二叉树:
1
/ \
2 3
/ \ \
4 5 6
\
7
*/
TreeNode *root = (TreeNode *)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode *)malloc(sizeof(TreeNode));
root->left->val = 2;
root->right = (TreeNode *)malloc(sizeof(TreeNode));
root->right->val = 3;
root->left->left = (TreeNode *)malloc(sizeof(TreeNode));
root->left->left->val = 4;
root->left->right = (TreeNode *)malloc(sizeof(TreeNode));
root->left->right->val = 5;
root->right->right = (TreeNode *)malloc(sizeof(TreeNode));
root->right->right->val = 6;
root->right->right->right = (TreeNode *)malloc(sizeof(TreeNode));
root->right->right->right->val = 7;
int width = getWidth(root, 4); // 计算二叉树深度为4时的宽度
printf("The width of the binary tree is: %d\n", width);
return 0;
}
```
阅读全文
相关推荐
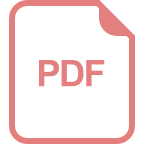
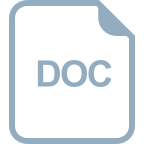






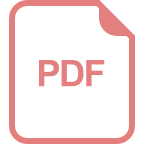
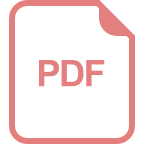





