在程序开始时用先序/中序/后序遍历的顺序建立二叉链表的递归算法 1、先序遍历二叉树的递归算法 2、中序遍历二叉树的递归算法 3、后序遍历二叉树的递归算法 4、计算二叉树的深度的递归算法 5、统计二叉树的结点个数的递归算法 6、统计二叉树的叶子结点个数的递归算法 7、设计该二叉树第K层的结点个数。 8、求该二叉树中所有结点值最大的元素。 9、打印二叉树的叶子结点数的递归算法(10分)
时间: 2023-11-13 18:25:19 浏览: 40
好的,我已经记录下来了。下面是对您提出的问题的回答:
1、先序遍历二叉树的递归算法:
```c++
void preOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
```
2、中序遍历二叉树的递归算法:
```c++
void inOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
```
3、后序遍历二叉树的递归算法:
```c++
void postOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
```
4、计算二叉树的深度的递归算法:
```c++
int depth(TreeNode* root) {
if (root == nullptr) {
return 0;
}
int leftDepth = depth(root->left);
int rightDepth = depth(root->right);
return max(leftDepth, rightDepth) + 1;
}
```
5、统计二叉树的结点个数的递归算法:
```c++
int countNodes(TreeNode* root) {
if (root == nullptr) {
return 0;
}
int leftCount = countNodes(root->left);
int rightCount = countNodes(root->right);
return leftCount + rightCount + 1;
}
```
6、统计二叉树的叶子结点个数的递归算法:
```c++
int countLeaves(TreeNode* root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
int leftLeaves = countLeaves(root->left);
int rightLeaves = countLeaves(root->right);
return leftLeaves + rightLeaves;
}
```
7、设计该二叉树第K层的结点个数:
```c++
int countNodesKthLevel(TreeNode* root, int k) {
if (root == nullptr) {
return 0;
}
if (k == 1) {
return 1;
}
int leftCount = countNodesKthLevel(root->left, k - 1);
int rightCount = countNodesKthLevel(root->right, k - 1);
return leftCount + rightCount;
}
```
8、求该二叉树中所有结点值最大的元素:
```c++
int findMax(TreeNode* root) {
if (root == nullptr) {
return INT_MIN;
}
int leftMax = findMax(root->left);
int rightMax = findMax(root->right);
return max(max(leftMax, rightMax), root->val);
}
```
9、打印二叉树的叶子结点数的递归算法:
```c++
void printLeaves(TreeNode* root) {
if (root == nullptr) {
return;
}
if (root->left == nullptr && root->right == nullptr) {
cout << root->val << " ";
return;
}
printLeaves(root->left);
printLeaves(root->right);
}
```
相关推荐
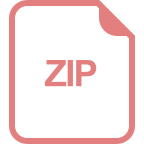















