设计一个 DealSentence(句子处理器) 类 ,包含:反向、分词、变大写的功能( 反向功能,可利用字符串的 ToCharArray()方法,然后数组用 Array 的成员函数 Reverse()来实现;分词功能,可利用字符串类的 Split()方法)
时间: 2024-09-20 13:16:05 浏览: 38
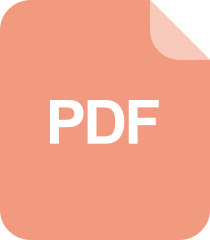
C#算法函数:获取一个字符串中的最大长度的数字
Designing a `DealSentence` class in Java can be accomplished by implementing the required methods for reversing a sentence, splitting it into words, and capitalizing each word. Here's an example of how you could structure this class:
```java
public class DealSentence {
private String sentence;
// 构造函数
public DealSentence(String sentence) {
this.sentence = sentence;
}
// 反转句子方法
public String reverse() {
char[] chars = sentence.toCharArray();
int start = 0;
int end = chars.length - 1;
while (start < end) {
char temp = chars[start];
chars[start] = chars[end];
chars[end] = temp;
start++;
end--;
}
return new String(chars);
}
// 分词方法
public String[] splitWords() {
return sentence.split(" ");
}
// 变大写方法,遍历每个单词并转换首字母为大写
public String[] toUpperCaseWords() {
String[] words = splitWords();
StringBuilder[] uppercasedWords = new StringBuilder[words.length];
for (int i = 0; i < words.length; i++) {
String word = words[i];
uppercasedWords[i] = new StringBuilder(word).toUpperCase().substring(0, 1) + word.substring(1);
}
return uppercasedWords;
}
}
```
使用这个类的例子:
```java
public static void main(String[] args) {
DealSentence processor = new DealSentence("Hello world, how are you?");
String reversed = processor.reverse(); // "you? are how world, Hello"
String[] splitWords = processor.splitWords(); // ["Hello", "world,", "how", "are", "you?"]
String[] uppedWords = processor.toUpperCaseWords(); // ["Hello", "WORLD,", "HOW", "ARE", "YOU?"]
// 打印结果
System.out.println("Reversed: " + reversed);
System.out.println("Split Words: " + Arrays.toString(splitWords));
System.out.println("Uppercase Words: " + Arrays.toString(uppedWords));
}
```
阅读全文
相关推荐
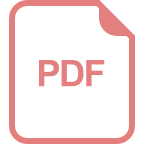
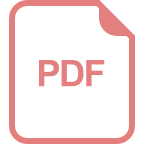
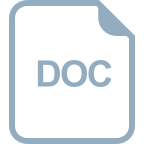














